rng.randint
时间: 2024-06-15 21:09:02 浏览: 102
rng.randint是一个用于生成随机整数的函数。rng是一个随机数生成器对象,而randint是该对象的一个方法。该方法接受两个参数,用于指定生成随机整数的范围。它会返回一个在指定范围内的随机整数。
例如,如果我们使用rng.randint(1, 10),它将会生成一个1到10之间(包括1和10)的随机整数。
相关问题
解释代码 indices = rng.randint(, len(y_pred), len(y_pred))
这段代码使用了 NumPy 库中的 randint 函数,用于生成随机整数。其中,第一个参数表示生成随机数的下界,第二个参数表示生成随机数的上界,第三个参数表示生成随机数的个数。在这段代码中,下界为 0,上界为 y_pred 数组的长度,生成的随机数个数也为 y_pred 数组的长度。生成的随机数将被用于对 y_pred 数组进行索引,从而随机选择 y_pred 数组中的元素。
将下列代码转换成python代码 #include <opencv2/opencv.hpp> #include <vector> #include <time.h> using namespace cv; using namespace std; // 8邻域 const Point neighbors[8] = { { 0, 1 }, { 1, 1 }, { 1, 0 }, { 1, -1 }, { 0, -1 }, { -1, -1 }, { -1, 0 }, {-1, 1} }; int main() { // 生成随机数 RNG rng(time(0)); Mat src = imread("1.jpg"); Mat gray; cvtColor(src, gray, CV_BGR2GRAY); Mat edges; Canny(gray, edges, 30, 100); vector<Point> seeds; vector<Point> contour; vector<vector<Point>> contours; int i, j, k; for (i = 0; i < edges.rows; i++) for (j = 0; j < edges.cols; j++) { Point c_pt = Point(i, j); //如果当前点为轮廓点 if (edges.at<uchar>(c_pt.x, c_pt.y) == 255) { contour.clear(); // 当前点清零 edges.at<uchar>(c_pt.x, c_pt.y) = 0; // 存入种子点及轮廓 seeds.push_back(c_pt); contour.push_back(c_pt); // 区域生长 while (seeds.size() > 0) { // 遍历8邻域 for (k = 0; k < 8; k++) { // 更新当前点坐标 c_pt.x = seeds[0].x + neighbors[k].x; c_pt.y = seeds[0].y + neighbors[k].y; // 边界界定 if ((c_pt.x >= 0) && (c_pt.x <= edges.rows - 1) && (c_pt.y >= 0) && (c_pt.y <= edges.cols - 1)) { if (edges.at<uchar>(c_pt.x, c_pt.y) == 255) { // 当前点清零 edges.at<uchar>(c_pt.x, c_pt.y) = 0; // 存入种子点及轮廓 seeds.push_back(c_pt); contour.push_back(c_pt); }// end if } } // end for // 删除第一个元素 seeds.erase(seeds.begin()); }// end while contours.push_back(contour); }// end if } // 显示一下 Mat trace_edge = Mat::zeros(edges.rows, edges.cols, CV_8UC1); Mat trace_edge_color; cvtColor(trace_edge, trace_edge_color, CV_GRAY2BGR); for (i = 0; i < contours.size(); i++) { Scalar color = Scalar(rng.uniform(0, 255), rng.uniform(0, 255), rng.uniform(0, 255)); //cout << edges[i].size() << endl; // 过滤掉较小的边缘 if (contours[i].size() > 5) { for (j = 0; j < contours[i].size(); j++) { trace_edge_color.at<Vec3b>(contours[i][j].x, contours[i][j].y)[0] = color[0]; trace_edge_color.at<Vec3b>(contours[i][j].x, contours[i][j].y)[1] = color[1]; trace_edge_color.at<Vec3b>(contours[i][j].x, contours[i][j].y)[2] = color[2]; } } } imshow("edge", trace_edge_color); waitKey(); return 0; }
```python
import cv2
import numpy as np
import random
# 8邻域
neighbors = [(0, 1), (1, 1), (1, 0), (1, -1), (0, -1), (-1, -1), (-1, 0), (-1, 1)]
# 读取图像
img = cv2.imread("1.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 30, 100)
seeds = []
contours = []
for i in range(edges.shape[0]):
for j in range(edges.shape[1]):
c_pt = (i, j)
# 如果当前点为轮廓点
if edges[c_pt] == 255:
contour = []
# 当前点清零
edges[c_pt] = 0
# 存入种子点及轮廓
seeds.append(c_pt)
contour.append(c_pt)
# 区域生长
while len(seeds) > 0:
for k in range(8):
# 更新当前点坐标
x = seeds[0][0] + neighbors[k][0]
y = seeds[0][1] + neighbors[k][1]
# 边界界定
if ((x >= 0) and (x <= edges.shape[0] - 1) and (y >= 0) and (y <= edges.shape[1] - 1)):
if edges[x, y] == 255:
# 当前点清零
edges[x, y] = 0
# 存入种子点及轮廓
seeds.append((x, y))
contour.append((x, y))
# 删除第一个元素
seeds.pop(0)
contours.append(contour)
# 显示一下
trace_edge = np.zeros((edges.shape[0], edges.shape[1], 1), dtype=np.uint8)
trace_edge_color = cv2.cvtColor(trace_edge, cv2.COLOR_GRAY2BGR)
for i in range(len(contours)):
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
# 过滤掉较小的边缘
if len(contours[i]) > 5:
for j in range(len(contours[i])):
trace_edge_color[contours[i][j][0], contours[i][j][1], 0] = color[0]
trace_edge_color[contours[i][j][0], contours[i][j][1], 1] = color[1]
trace_edge_color[contours[i][j][0], contours[i][j][1], 2] = color[2]
cv2.imshow("edge", trace_edge_color)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
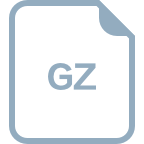
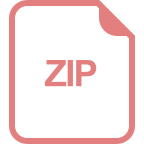
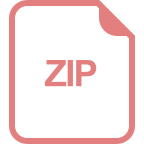
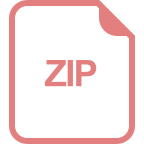
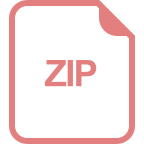
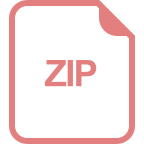
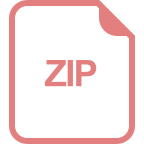
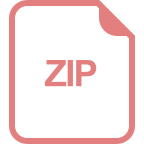
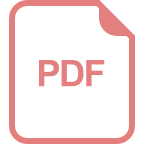
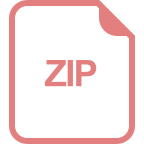
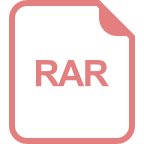




