python凯撒密码加密解密
时间: 2023-07-25 18:14:47 浏览: 175
以下是Python实现凯撒密码加密解密的代码:
```python
def encrypt_caesar(plaintext, shift):
"""
凯撒密码加密函数
:param plaintext: 明文字符串
:param shift: 偏移量,即密钥
:return: 密文字符串
"""
ciphertext = ''
for char in plaintext:
if char.isalpha():
# 将字母转换为 ASCII 码值
char_code = ord(char)
# 判断字母是大写还是小写
if char.isupper():
# 对大写字母进行加密
char_code = (char_code - 65 + shift) % 26 + 65
else:
# 对小写字母进行加密
char_code = (char_code - 97 + shift) % 26 + 97
# 将加密后的 ASCII 码值转换回字母
char = chr(char_code)
# 将加密后的字符添加到密文字符串中
ciphertext += char
return ciphertext
def decrypt_caesar(ciphertext, shift):
"""
凯撒密码解密函数
:param ciphertext: 密文字符串
:param shift: 偏移量,即密钥
:return: 明文字符串
"""
plaintext = ''
for char in ciphertext:
if char.isalpha():
# 将字母转换为 ASCII 码值
char_code = ord(char)
# 判断字母是大写还是小写
if char.isupper():
# 对大写字母进行解密
char_code = (char_code - 65 - shift) % 26 + 65
else:
# 对小写字母进行解密
char_code = (char_code - 97 - shift) % 26 + 97
# 将解密后的 ASCII 码值转换回字母
char = chr(char_code)
# 将解密后的字符添加到明文字符串中
plaintext += char
return plaintext
```
其中,`encrypt_caesar`函数用于加密明文字符串,`decrypt_caesar`函数用于解密密文字符串。两个函数的参数相同,分别为明文或密文字符串和偏移量(即密钥)。函数的实现过程是:
1. 遍历明文或密文字符串中的每个字符。
2. 如果字符是字母,则将其转换为 ASCII 码值。
3. 判断字母是大写字母还是小写字母,对其进行加密或解密。
4. 将加密或解密后的 ASCII 码值转换回字母,并将其添加到密文或明文字符串中。
5. 返回加密或解密后的密文或明文字符串。
示例:
```python
plaintext = 'hello world'
shift = 3
ciphertext = encrypt_caesar(plaintext, shift)
print(ciphertext) # khoor zruog
decrypted_plaintext = decrypt_caesar(ciphertext, shift)
print(decrypted_plaintext) # hello world
```
相关推荐
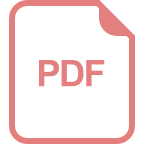
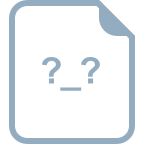
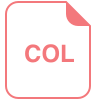
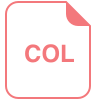











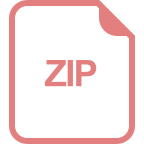
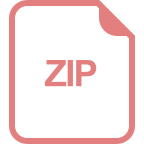