输出一个完整vue增删改查的代码
时间: 2023-11-22 22:55:23 浏览: 76
以下是一个简单的Vue增删改查的代码示例:
```html
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Vue CRUD Example</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
</head>
<body>
<div id="app">
<h2>Vue CRUD Example</h2>
<div>
<label>Name:</label>
<input type="text" v-model="newItem.name">
<button @click="addItem">Add</button>
</div>
<table>
<thead>
<tr>
<th>Name</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in items">
<td>{{ item.name }}</td>
<td>
<button @click="editItem(index)">Edit</button>
<button @click="deleteItem(index)">Delete</button>
</td>
</tr>
</tbody>
</table>
<div v-if="selectedItem !== null">
<h3>Edit Item</h3>
<div>
<label>Name:</label>
<input type="text" v-model="selectedItem.name">
<button @click="updateItem">Update</button>
<button @click="cancelEdit">Cancel</button>
</div>
</div>
</div>
<script src="js/app.js"></script>
</body>
</html>
```
```javascript
// app.js
new Vue({
el: "#app",
data: {
items: [],
newItem: {
name: ""
},
selectedItem: null
},
mounted() {
this.getItems();
},
methods: {
getItems() {
axios.get("/api/items")
.then(response => {
this.items = response.data;
})
.catch(error => {
console.log(error);
});
},
addItem() {
axios.post("/api/items", this.newItem)
.then(response => {
this.items.push(response.data);
this.newItem.name = "";
})
.catch(error => {
console.log(error);
});
},
editItem(index) {
this.selectedItem = this.items[index];
},
updateItem() {
axios.put(`/api/items/${this.selectedItem.id}`, this.selectedItem)
.then(response => {
this.selectedItem = null;
})
.catch(error => {
console.log(error);
});
},
deleteItem(index) {
const id = this.items[index].id;
axios.delete(`/api/items/${id}`)
.then(response => {
this.items.splice(index, 1);
})
.catch(error => {
console.log(error);
});
},
cancelEdit() {
this.selectedItem = null;
}
}
});
```
这个例子使用了Vue.js和Axios来实现增删改查的功能,同时通过RESTful API来与后端进行交互。你需要在后端实现以下API:
- GET /api/items:获取所有items
- POST /api/items:添加一个item
- PUT /api/items/:id:更新一个item
- DELETE /api/items/:id:删除一个item
这个例子中,我们使用了一个简单的数组来存储items,你可以根据你的需要将其替换为真正的数据库。
阅读全文
相关推荐

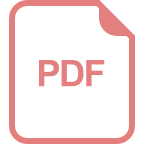
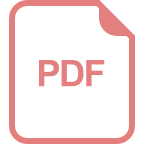
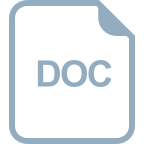
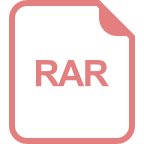
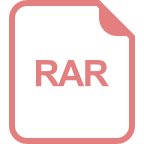
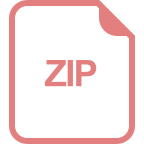
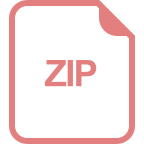
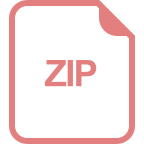
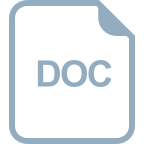
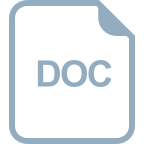
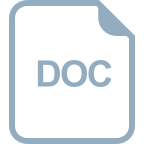
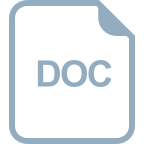
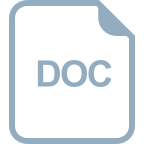
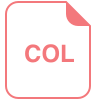