python怎么统计列表中元素出现的次数并排序
时间: 2023-06-08 21:05:56 浏览: 66
可以使用Python内置的Counter类来统计列表中元素出现次数,并使用sorted函数对结果进行排序。示例代码如下:
```
from collections import Counter
lst = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5]
count = Counter(lst)
result = sorted(count.items(), key=lambda x: x[1], reverse=True)
print(result)
```
输出结果为:
```
[(4, 4), (3, 3), (2, 2), (5, 2), (1, 1)]
```
其中,每个元素为一个元组,包含元素本身和出现次数。可以根据需要进行进一步处理。
相关问题
python统计列表中各个元素出现的次数
可以使用 python 内置的 `collections` 模块中的 `Counter` 类来统计列表中各个元素出现的次数。举个例子:
```
from collections import Counter
my_list = [1, 2, 3, 2, 1, 3, 1, 2, 3]
counts = Counter(my_list)
print(counts)
```
输出:
```
Counter({1: 3, 2: 3, 3: 3})
```
可以看到,它会将元素和出现次数封装为一个字典返回。
也可以统计其他类型的数据,例如字符串
```
string = 'hello world'
counts = Counter(string)
print(counts)
```
输出
```
Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1})
```
如果要获取每个元素出现次数最多的 n 个元素,可以使用 `most_common()` 方法,例如:
```
counts.most_common(2)
```
输出:
```
[(1, 3), (2, 3)]
```
需要注意的是,如果数据量很大,那么在统计前先排序是更加高效的。
如果你的数据类型比较特殊,那么需要额外考虑使用hash_map或者hash_set之类的数据结构。
python 根据数组中元素的出现次数进行排序
在Python中,可以使用collections模块中的Counter类来计算数组中元素的出现次数,并使用sorted函数对结果进行排序。
首先,我们需要导入collections模块:
```python
import collections
```
然后,可以使用Counter类对数组中的元素进行计数:
```python
arr = [1, 3, 2, 2, 3, 1, 1, 4]
counter = collections.Counter(arr)
```
接下来,可以使用sorted函数对counter对象的元素进行排序,按照出现次数从小到大排序。为了实现这个功能,我们可以利用sorted函数的key参数,指定排序的依据:
```python
sorted_arr = sorted(counter.items(), key=lambda x: x[1])
```
在这里,我们使用了lambda函数来定义排序依据。x[1]表示counter对象中每个元素的出现次数。这样,sorted_arr就是按照元素的出现次数进行排序后的数组。
完整的代码如下:
```python
import collections
arr = [1, 3, 2, 2, 3, 1, 1, 4]
counter = collections.Counter(arr)
sorted_arr = sorted(counter.items(), key=lambda x: x[1])
```
这样,sorted_arr就是按照数组中元素的出现次数进行排序的结果。
相关推荐
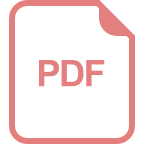
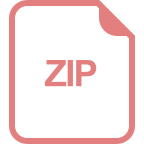












