使用c语言编写字符定位,输入一个字符串和一个字符,如果该字符在字符串中,就从该字符首次出现的位置开始输出字符串中的字符。本题要求编写函数(s,ch),在字符串S中查找字符ch,如果找到,返回第一次找到的该字符在字符串中的位置(地址);否则,返回空指针NULL
时间: 2024-10-24 14:17:26 浏览: 10
在C语言中,你可以编写一个名为`find_char_pos`的函数来实现这个功能。这个函数接受两个参数,一个是字符串`s`,另一个是要查找的字符`ch`。下面是一个简单的实现示例:
```c
#include <stdio.h>
#include <string.h>
// 定义函数 find_char_pos
char* find_char_pos(const char* s, char ch) {
// 首先检查字符串是否为空
if (s == NULL) {
return NULL;
}
// 使用 while 循环遍历字符串,寻找字符ch的位置
int index = 0;
while (s[index] != '\0') { // '\0' 表示字符串结束
if (s[index] == ch) {
// 如果找到字符,记录位置并返回非空指针
return (char*) &s[index]; // 返回字符的地址
}
index++;
}
// 如果整个字符串都遍历完了还没找到字符,返回 NULL
return NULL;
}
int main() {
const char* str = "Hello, World!";
char ch = 'o';
char* pos = find_char_pos(str, ch);
if (pos != NULL) {
printf("Character '%c' found at position %d\n", ch, pos - str); // 减去str是为了获取索引而不是地址
} else {
printf("Character '%c' not found in the string.\n", ch);
}
return 0;
}
```
在这个例子中,我们首先检查字符串是否为空,然后遍历每个字符,当找到目标字符时返回其地址。如果没有找到,函数会返回NULL。
阅读全文
相关推荐
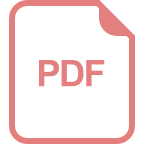
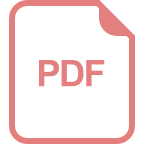
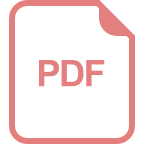
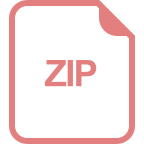
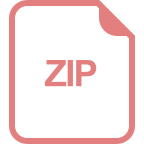
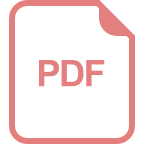
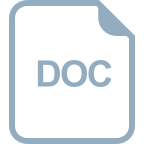
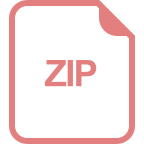
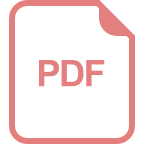
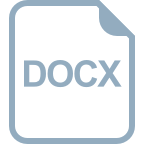
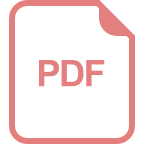