java RSA加密工具类编写
时间: 2024-02-24 12:36:08 浏览: 96
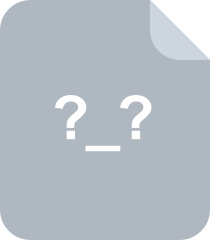
JAVA-RSA加密工具类
以下是一个简单的Java RSA加密工具类的代码示例:
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import javax.crypto.Cipher;
public class RSAUtils {
// 生成密钥对
public static KeyPair generateKeyPair() throws NoSuchAlgorithmException {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
SecureRandom secureRandom = new SecureRandom();
keyPairGenerator.initialize(2048, secureRandom); // 密钥长度为2048位
return keyPairGenerator.generateKeyPair();
}
// 加密
public static byte[] encrypt(byte[] input, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(input);
}
// 解密
public static byte[] decrypt(byte[] input, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(input);
}
}
使用方法:
// 生成密钥对
KeyPair keyPair = RSAUtils.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 加密
byte[] data = "Hello, world!".getBytes();
byte[] encrypted = RSAUtils.encrypt(data, publicKey);
// 解密
byte[] decrypted = RSAUtils.decrypt(encrypted, privateKey);
String message = new String(decrypted);
System.out.println(message); // 输出:Hello, world!
阅读全文
相关推荐
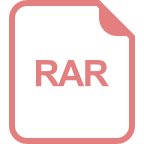
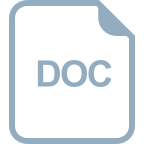
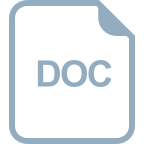
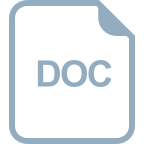
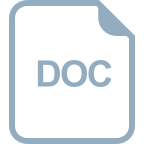
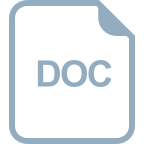
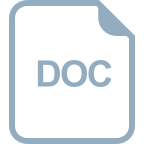
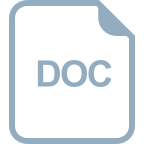
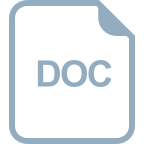
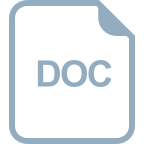
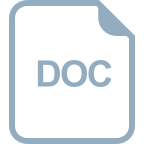
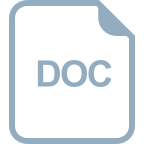
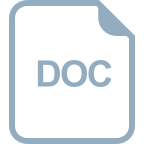
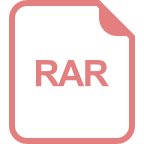
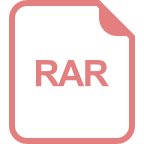
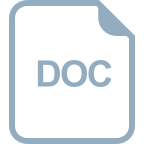