c语言实现通讯录(CGI)详细代码
时间: 2023-10-19 20:05:14 浏览: 245
下面是一个简单的C语言实现通讯录的CGI程序的代码,具体实现方式可能因实际需求不同而有所差异:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CONTACTS 1000
#define MAX_NAME_LEN 50
#define MAX_PHONE_LEN 20
#define MAX_ADDR_LEN 100
typedef struct {
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
char addr[MAX_ADDR_LEN];
} Contact;
Contact contacts[MAX_CONTACTS];
int num_contacts = 0;
void load_contacts() {
FILE *fp = fopen("contacts.csv", "r");
if (fp == NULL) {
return;
}
char line[256];
while (fgets(line, sizeof(line), fp) != NULL) {
char *name = strtok(line, ",");
char *phone = strtok(NULL, ",");
char *addr = strtok(NULL, ",");
if (name != NULL && phone != NULL && addr != NULL) {
Contact c;
strncpy(c.name, name, MAX_NAME_LEN);
strncpy(c.phone, phone, MAX_PHONE_LEN);
strncpy(c.addr, addr, MAX_ADDR_LEN);
contacts[num_contacts++] = c;
}
}
fclose(fp);
}
void save_contacts() {
FILE *fp = fopen("contacts.csv", "w");
if (fp == NULL) {
return;
}
for (int i = 0; i < num_contacts; i++) {
fprintf(fp, "%s,%s,%s\n", contacts[i].name, contacts[i].phone, contacts[i].addr);
}
fclose(fp);
}
void add_contact(const char *name, const char *phone, const char *addr) {
if (num_contacts >= MAX_CONTACTS) {
return;
}
Contact c;
strncpy(c.name, name, MAX_NAME_LEN);
strncpy(c.phone, phone, MAX_PHONE_LEN);
strncpy(c.addr, addr, MAX_ADDR_LEN);
contacts[num_contacts++] = c;
save_contacts();
}
void delete_contact(const char *name) {
for (int i = 0; i < num_contacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
for (int j = i; j < num_contacts - 1; j++) {
contacts[j] = contacts[j + 1];
}
num_contacts--;
save_contacts();
break;
}
}
}
void modify_contact(const char *name, const char *phone, const char *addr) {
for (int i = 0; i < num_contacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
strncpy(contacts[i].phone, phone, MAX_PHONE_LEN);
strncpy(contacts[i].addr, addr, MAX_ADDR_LEN);
save_contacts();
break;
}
}
}
void search_contact(const char *name, char *phone, char *addr) {
for (int i = 0; i < num_contacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
strncpy(phone, contacts[i].phone, MAX_PHONE_LEN);
strncpy(addr, contacts[i].addr, MAX_ADDR_LEN);
break;
}
}
}
int main() {
load_contacts();
char *query = getenv("QUERY_STRING");
if (query == NULL) {
printf("Content-type:text/html\n\n");
printf("<html><body>");
printf("<h1>Contact Book</h1>");
printf("<form method=\"post\">");
printf("<p>Name: <input type=\"text\" name=\"name\"></p>");
printf("<p>Phone: <input type=\"text\" name=\"phone\"></p>");
printf("<p>Address: <input type=\"text\" name=\"addr\"></p>");
printf("<p><input type=\"submit\" value=\"Add\" name=\"action\">");
printf("<input type=\"submit\" value=\"Delete\" name=\"action\">");
printf("<input type=\"submit\" value=\"Modify\" name=\"action\">");
printf("<input type=\"submit\" value=\"Search\" name=\"action\"></p>");
printf("</form>");
printf("</body></html>");
} else {
char name[MAX_NAME_LEN], phone[MAX_PHONE_LEN], addr[MAX_ADDR_LEN];
sscanf(query, "name=%[^&]&phone=%[^&]&addr=%[^&]", name, phone, addr);
char *action = strstr(query, "action=");
if (action == NULL) {
printf("Content-type:text/html\n\n");
printf("<html><body>");
printf("<h1>Contact Book</h1>");
printf("<p>Invalid action.</p>");
printf("</body></html>");
} else if (strncmp(action + 7, "Add", 3) == 0) {
add_contact(name, phone, addr);
printf("Location: /cgi-bin/contact.cgi\n\n");
} else if (strncmp(action + 7, "Delete", 6) == 0) {
delete_contact(name);
printf("Location: /cgi-bin/contact.cgi\n\n");
} else if (strncmp(action + 7, "Modify", 6) == 0) {
modify_contact(name, phone, addr);
printf("Location: /cgi-bin/contact.cgi\n\n");
} else if (strncmp(action + 7, "Search", 6) == 0) {
char phone[MAX_PHONE_LEN], addr[MAX_ADDR_LEN];
search_contact(name, phone, addr);
printf("Content-type:text/html\n\n");
printf("<html><body>");
printf("<h1>Contact Book</h1>");
printf("<p>Phone: %s</p>", phone);
printf("<p>Address: %s</p>", addr);
printf("</body></html>");
} else {
printf("Content-type:text/html\n\n");
printf("<html><body>");
printf("<h1>Contact Book</h1>");
printf("<p>Invalid action.</p>");
printf("</body></html>");
}
}
return 0;
}
```
以上代码实现了通讯录的添加、删除、修改、查询联系人等功能,使用文件存储通讯录。注意代码中的安全性验证和文件读写权限等细节。
阅读全文
相关推荐
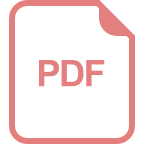
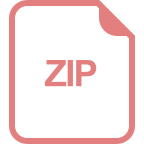




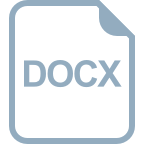
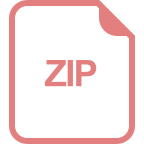

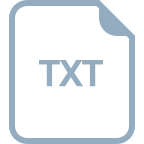
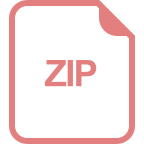