for textbox_child in textbox.iterdescendants()
时间: 2023-06-16 17:06:27 浏览: 74
这段代码是在使用一个名为 `textbox` 的对象的方法 `iterdescendants()` 进行迭代。`iterdescendants()` 方法返回 `textbox` 对象的所有子孙元素,包括它自己。这个代码块使用一个 `for` 循环来遍历所有子孙元素,并将每个元素赋值给变量 `textbox_child`。在循环体内,我们可以对 `textbox_child` 进行操作。
相关问题
private void TextBox_Project_Name(object sender, TextChangedEventArgs e) { string Project_Name = textBox1.Text; Console.WriteLine(Project_Name); }这段代码有什么错误?
这段代码的错误在于事件的命名不正确,应该是"TextChanged"而不是"TextChangedEventArgs"。正确的代码应该是:
```
private void TextBox_Project_Name(object sender, TextChangedEventArgs e)
{
string Project_Name = textBox1.Text;
Console.WriteLine(Project_Name);
}
```
另外,您还需要确保已将事件绑定到文本框控件上。可以在窗体的构造函数中添加以下代码来完成绑定:
```
public Form1()
{
InitializeComponent();
textBox1.TextChanged += TextBox_Project_Name;
}
```
这样,每当文本框的文本内容改变时,TextChanged事件就会被触发,并且执行TextBox_Project_Name方法,将文本框中的文本内容输出到控制台。
ini.IniWriteValue("光晕标准", "半径上限", banjingshangxian_textBox.Text); ini.IniWriteValue("光晕标准", "半径下限", banjingxiaxian_textBox.Text); ini.IniWriteValue("光晕标准", "缺口上限", quekou_max_textBox.Text); ini.IniWriteValue("光晕标准", "偏心上限", pianxin_max_textBox.Text); ini.IniWriteValue("光晕标准", "黑点上限", heidian_max_textBox.Text); ini.IniWriteValue("光晕标准", "内圆圆度上限", neiyuanyuandu_max_textBox.Text);
这段代码是用来写入INI配置文件的。具体来说,它将一些参数值写入了一个叫做“光晕标准”的section中,包括半径上限、半径下限、缺口上限、偏心上限、黑点上限和内圆圆度上限。这些参数可能是用来控制某个程序或者系统的一些行为或者属性的。INI配置文件是一种常见的存储配置信息的格式,可以被很多程序和系统所使用。
相关推荐
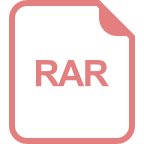
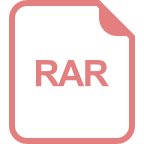
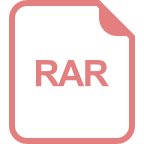
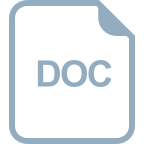
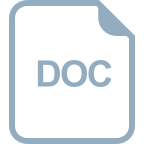
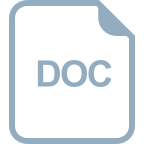
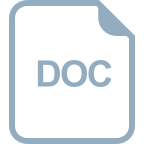
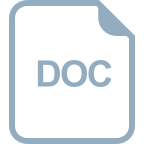








