def select_file(self):
时间: 2024-01-27 14:04:58 浏览: 111
这是一个方法,用于让用户选择一个文件。具体实现可以根据你的应用场景来选择使用哪种方式,比如使用系统对话框、命令行参数等。以下是一个示例代码:
```python
import tkinter as tk
from tkinter import filedialog
class FileSelector:
def __init__(self):
self.root = tk.Tk()
self.root.withdraw()
def select_file(self, title="Select File", filetypes=(("all files", "*.*"),)):
file_path = filedialog.askopenfilename(title=title, filetypes=filetypes)
return file_path
```
这是一个使用 tkinter 库实现的图形界面,通过 `filedialog.askopenfilename` 方法打开系统对话框来选择文件。调用方法如下:
```python
file_selector = FileSelector()
file_path = file_selector.select_file()
print(file_path)
```
相关问题
下面这段代码的作用是什么: def __init__(self, title): super().__init__() self.ui = Ui_Dialog() self.ui.setupUi(self) self.save_path = 'result' self.save_id = 0 if not os.path.exists(self.save_path): os.mkdir(self.save_path) self.now = None self.model = None self.video_count = None self._timer = None self.ui.textBrowser.setFontPointSize(18) self.ui.label.setText(title) self.ui.pushButton_Model.clicked.connect(self.select_model) self.ui.pushButton_Img.clicked.connect(self.select_image_file) self.ui.pushButton_ImgFolder.clicked.connect(self.select_folder_file) self.ui.pushButton_Video.clicked.connect(self.select_video_file) self.ui.pushButton_Camera.clicked.connect(self.select_camear) self.ui.pushButton_SavePath.clicked.connect(self.select_savepath) self.ui.pushButton_BegDet.clicked.connect(self.begin_detect) self.ui.pushButton_StopDet.clicked.connect(self.stop_detect) self.ui.pushButton_Exit.clicked.connect(self._exit) self.show()
这段代码的作用是初始化一个名为 "title" 的类,并且连接了多个按钮的点击事件,用于选择模型、选择文件、选择摄像头、选择保存路径等操作。同时创建了一个名为 "result" 的文件夹,用于存储检测结果。
import tkinter as tk from tkinter import filedialog import pyautogui class Application(tk.Frame): def __init__(self, master=None): super().__init__(master) self.master = master self.pack() self.create_widgets() def create_widgets(self): self.select_button = tk.Button(self) self.select_button["text"] = "选择图片" self.select_button["command"] = self.select_image self.select_button.pack(side="top") self.result_label = tk.Label(self) self.result_label.pack(side="bottom") def select_image(self): # 弹出文件选择对话框选择要查找的图片 file_path = filedialog.askopenfilename(title="选择图片文件", filetypes=[("Image files", "*.png;*.jpg;*.jpeg")]) # 如果选择了图片则查找图片位置 if file_path: location = find_image_location(file_path) if location is not None: self.result_label["text"] = f"图片 {file_path} 的位置坐标为:{location}" else: self.result_label["text"] = f"图片 {file_path} 不存在于桌面" def find_image_location(image_path): # 加载图片并获取图片位置坐标 location = pyautogui.locateOnScreen(image_path) if location is not None: # 获取图片中心点的坐标 x, y = pyautogui.center(location) return (x, y) else: return None root = tk.Tk() app = Application(master=root) app.mainloop()
这段代码是一个 Python GUI 应用程序,它使用 tkinter 模块来创建图形界面。应用程序的主要功能是选择图片文件并在桌面上查找该图片的位置坐标。
应用程序的主类是 Application,它继承自 tkinter.Frame 类。在 __init__ 方法中,它创建了一个顶层窗口 master,并将自身显示在窗口上。在 create_widgets 方法中,它创建了一个按钮和一个标签,并将它们放在窗口上。当用户点击按钮时,它会调用 select_image 方法来选择要查找位置的图片文件。
select_image 方法中,它使用 filedialog 模块弹出一个文件选择对话框,让用户选择要查找位置的图片文件。如果用户选择了图片文件,则调用 find_image_location 方法来查找该图片的位置坐标。如果找到了该图片,则在标签上显示该图片的位置坐标;否则,在标签上显示该图片不存在于桌面。
find_image_location 方法使用 pyautogui 模块来查找图片在屏幕上的位置。它加载图片文件并使用 locateOnScreen 方法来查找该图片在屏幕上的位置。如果找到了该图片,则使用 center 方法来获取图片中心点的坐标,并返回该坐标;否则返回 None。
最后,在主程序中创建一个 Application 实例,并调用主循环来运行应用程序。
阅读全文
相关推荐
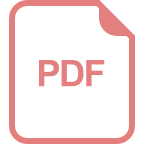
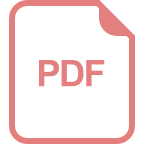







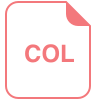
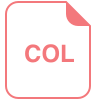
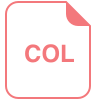




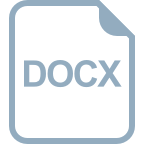