给定一个数字列表[3,1,4,1,5,9,2,6]并对其进行排序
时间: 2024-11-09 12:17:26 浏览: 11
给定一个数字列表 `[3, 1, 4, 1, 5, 9, 2, 6]`,你可以使用Python内置的`sort()`函数或者`sorted()`函数对它进行排序。这里分别展示两种方法:
1. 使用`sort()`函数原地排序:
```python
numbers = [3, 1, 4, 1, 5, 9, 2, 6]
numbers.sort()
```
之后,`numbers` 就会被改变为 `[1, 1, 2, 3, 4, 5, 6, 9]`。
2. 使用`sorted()`函数创建一个新的排序后的列表:
```python
numbers = [3, 1, 4, 1, 5, 9, 2, 6]
sorted_numbers = sorted(numbers)
```
`sorted_numbers` 会是一个新的列表 `[1, 1, 2, 3, 4, 5, 6, 9]`,原始列表`numbers`保持不变。
如果你需要按照降序排列,可以传入 `reverse=True` 参数:
```python
sorted_numbers_desc = sorted(numbers, reverse=True) # 或者 numbers.sort(reverse=True)
```
这将得到 `[9, 6, 5, 4, 3, 2, 1, 1]`。
阅读全文
相关推荐
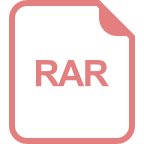
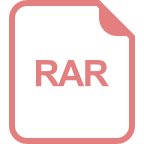
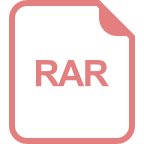
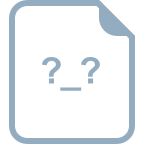
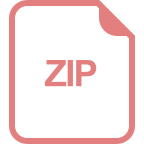
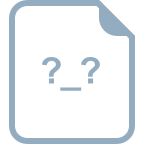
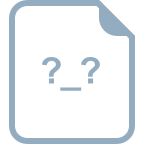
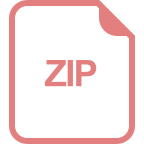
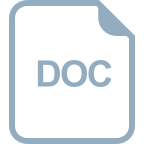
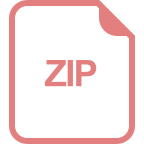
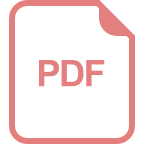
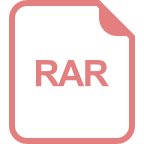
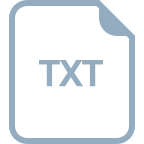
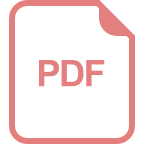
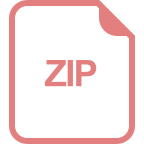
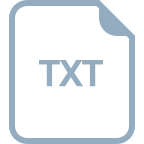
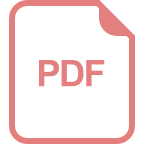
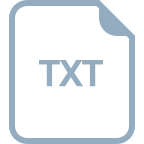
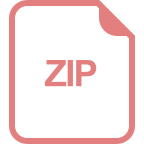