如何使用Python实现一个多线程的ICMP扫描程序来检测网络上运行的计算机,并解析返回结果并创建一个图形用户界面展示这些信息?
时间: 2024-10-28 08:15:33 浏览: 32
要在Python中实现一个多线程的ICMP扫描程序,你可以使用`threading`模块配合`socket`和`icmp`模块(如果使用第三方库如`scapy`的话)。以下是一个简单的示例:
```python
import socket
from threading import Thread
from queue import Queue
def icmp_scan(hosts, results_queue):
for host in hosts:
sock = socket.socket(socket.AF_INET, socket.SOCK_RAW, socket.IPPROTO_ICMP)
try:
response = sock.sendto(b'ping', (host, 0))
data, addr = sock.recvfrom(512)
if 'echo reply' in str(data): # 检查是否有Echo Reply
results_queue.put((host, 'online'))
else:
results_queue.put((host, 'offline'))
except Exception as e:
results_queue.put((host, f'error: {e}'))
if __name__ == "__main__":
hosts_to_scan = ['192.168.1.1', '192.168.1.2', ...] # 需要扫描的IP列表
results_queue = Queue()
threads = []
for host in hosts_to_scan:
t = Thread(target=icmp_scan, args=(host, results_queue))
threads.append(t)
t.start()
# 等待所有线程完成
for t in threads:
t.join()
while not results_queue.empty():
host, status = results_queue.get()
print(f"{host}: {status}")
# 创建简单GUI(如使用Tkinter)
from tkinter import Tk, Label
root = Tk()
for host, status in results.items(): # 假设results是从queue得到的结果字典
Label(root, text=f"{host}: {status}").grid(row=len(results)//4, column=0)
root.mainloop()
```
这个脚本首先启动多个线程对指定IP地址进行ICMP ping扫描,然后将结果存入队列。主线程等待所有扫描完成后,取出队列中的结果并在控制台显示。最后,使用基本的Tkinter界面显示每个主机的状态。
阅读全文
相关推荐
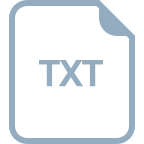
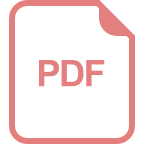
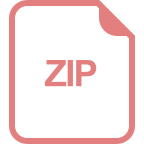
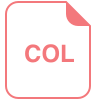
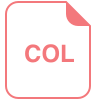
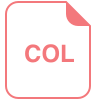
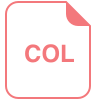
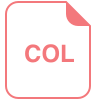
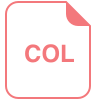
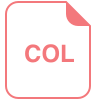
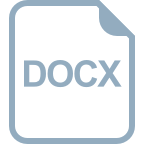
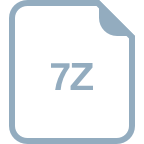
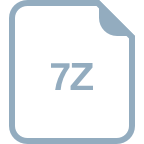
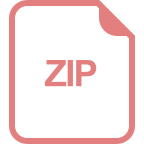
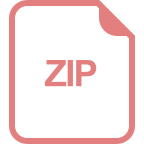