write a C++ program for constructing a heap (max heap) with integers and printing it in the rotated form.
时间: 2024-02-29 18:52:13 浏览: 84
Sure, here's the code:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void heapify(vector<int>& arr, int n, int i) {
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && arr[l] > arr[largest])
largest = l;
if (r < n && arr[r] > arr[largest])
largest = r;
if (largest != i) {
swap(arr[i], arr[largest]);
heapify(arr, n, largest);
}
}
void buildHeap(vector<int>& arr) {
int n = arr.size();
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
}
void printHeap(vector<int>& arr) {
int n = arr.size();
int row = 1, col = 0;
while (col < n) {
for (int i = col; i < min(n, col + row); i++)
cout << arr[i] << " ";
cout << endl;
col += row;
row *= 2;
}
}
int main() {
vector<int> arr = { 4, 10, 3, 5, 1 };
buildHeap(arr);
printHeap(arr);
}
```
This program first constructs a max heap from a given vector of integers using the `buildHeap` function. It then prints the heap in a rotated format using the `printHeap` function.
The `heapify` function is used to maintain the heap property while constructing the heap. It takes an array `arr`, its size `n`, and the index of the current node `i` as input. It compares the value at the current node with its left and right child nodes, and swaps the current node with the largest child node if necessary. It then recursively calls itself on the largest child node to maintain the heap property.
The `buildHeap` function iterates over the second half of the array in reverse order (since these nodes are the ones with children) and calls `heapify` on each node to construct the heap.
The `printHeap` function prints the heap in a rotated format. It first initializes the row and column numbers, and then iterates over each row of the heap, printing the nodes in that row and updating the column and row numbers accordingly.
Note that this program assumes a complete binary tree representation of the heap. If the input vector is not complete, the program may produce unexpected results.
阅读全文
相关推荐
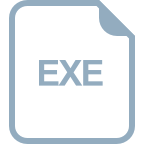

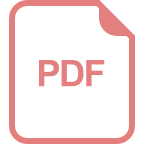
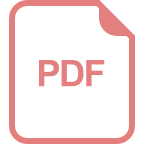
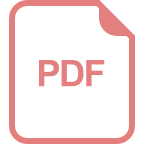
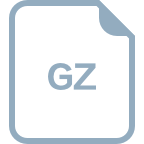
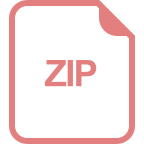
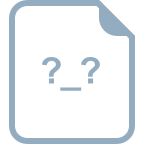
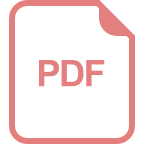
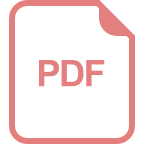
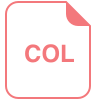




