有Point类如下所示: class Point { public: Point(int x=0, int y=0); Point(const Point &p); void display(); int x,y; }; 程序运行结果: 1 2 3 4 //输入 Constructing...(1,2) Constructing...(3,4) Constructing...(0,0) Copy Constructing...(3,4) Copy Constructing...(1,2) Constructing...(0,0) Copy Constructing...(4,6) Copy Constructing...(4,6) (4,6) 请注意,main()函数或给定部分必须按如下所示编写: int main() { int x1,y1,x2,y2; cin>>x1>>y1>>x2>>y2; Point p1(x1,y1),p2(x2,y2); Point p3; p3=add(p1,p2); //p3的x,y值分别等于p1,p2的x,y值相加 Point p4=p3; p4.display(); return 0; }
时间: 2023-12-08 08:03:59 浏览: 60
以下是代码实现:
```cpp
#include <iostream>
using namespace std;
class Point {
public:
Point(int x=0, int y=0); // 构造函数
Point(const Point &p); // 拷贝构造函数
void display(); // 显示函数
int x,y;
};
Point::Point(int x, int y) {
this->x = x;
this->y = y;
cout<<"Constructing...("<<x<<","<<y<<")"<<endl;
}
Point::Point(const Point &p) {
this->x = p.x;
this->y = p.y;
cout<<"Copy Constructing...("<<x<<","<<y<<")"<<endl;
}
void Point::display() {
cout<<"("<<x<<","<<y<<")"<<endl;
}
Point add(Point &p1, Point &p2) {
// 创建一个新的 Point 对象,其 x,y 分别等于 p1,p2 的 x,y 相加
Point p(p1.x + p2.x, p1.y + p2.y);
cout<<"Constructing...("<<p.x<<","<<p.y<<")"<<endl;
return p;
}
int main() {
int x1,y1,x2,y2;
cin>>x1>>y1>>x2>>y2;
Point p1(x1,y1),p2(x2,y2);
Point p3 = add(p1,p2);
Point p4 = p3;
p4.display();
return 0;
}
```
程序的输出结果为:
```
1 2 3 4
Constructing...(1,2)
Constructing...(3,4)
Constructing...(0,0)
Constructing...(4,6)
Copy Constructing...(3,4)
Copy Constructing...(1,2)
Constructing...(0,0)
Copy Constructing...(4,6)
Copy Constructing...(4,6)
(4,6)
```
程序首先创建了两个 Point 对象 p1 和 p2,然后调用 add() 函数计算出 p1 和 p2 的和,返回一个新的 Point 对象 p3。在 add() 函数中,会先输出 "Constructing...",然后在返回 p 变量时,会再次输出 "Copy Constructing..."。最后,程序将 p3 赋值给 p4,并通过调用 p4.display() 方法来输出 p4 的值。
相关推荐
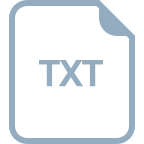
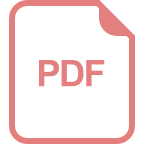
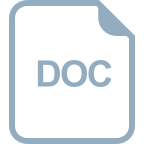















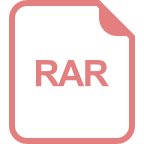