如何用c语言将字符串中的小写字母改为大写字母
时间: 2024-10-21 07:05:07 浏览: 17
在C语言中,你可以通过标准库函数`toupper()`来将小写字母转换为大写字母。这里是一个简单的示例,展示如何遍历一个字符串并转换其中的小写字母:
```c
#include <ctype.h> // 包含 toupper 函数
#include <stdio.h>
void convert_to_upper(char* str) {
for(int i = 0; str[i] != '\0'; i++) { // 遍历字符串直到遇到结束符'\0'
if(islower(str[i])) { // 检查字符是否为小写字母
str[i] = toupper(str[i]); // 转换为大写
}
}
}
int main() {
char small_str[] = "Hello, world!";
convert_to_upper(small_str);
printf("转换后的字符串: %s\n", small_str); // 输出 "HELLO, WORLD!"
return 0;
}
```
在这个例子中,`islower()`函数用于检查字符是否为小写字母,`toupper()`函数则负责转换字符。
相关问题
如何用C语言将字符串中的小写字母转换为大写字母?
在C语言中,你可以使用`ctype.h`库提供的`toupper()`函数将小写字母转换为大写字母。以下是一个简单的步骤:
1. 包含头文件:首先,需要包含`ctype.h`头文件,它包含了字符处理相关的函数声明。
```c
#include <ctype.h>
```
2. 使用 toupper() 函数:遍历输入的字符串,对于每个字符检查是否是小写字母,如果是,则调用 `toupper()` 函数将其转换为大写。
```c
char *to_upper_case(char *str) {
int i = 0;
while (str[i] != '\0') { // 遍历直到遇到字符串结束符'\0'
if (islower(str[i])) { // 检查字符是否为小写
str[i] = toupper(str[i]); // 如果是,转换为大写
}
i++;
}
return str; // 返回转换后的字符串
}
```
3. 示例:
```c
int main() {
char input[] = "hello world";
printf("Original string: %s\n", input);
input = to_upper_case(input); // 调用转换函数
printf("Converted string: %s\n", input);
return 0;
}
```
运行上面的例子,原始字符串 "hello world" 将会变成 "HELLO WORLD"。
c语言讲字符串中小写字母转换成大写用while
循环实现的方法是什么?
答案:可以使用 while 循环以及条件判断实现小写字母到大写字母的转换。具体实现过程可以先使用 C 语言字符串函数 strlen 计算字符串长度,然后使用 toupper 函数将小写字母转换为大写字母,最后将结果存储到原字符串中。以下是示例代码:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int main() {
char str[100];
int i = 0, len;
printf("请输入字符串: ");
scanf("%s", str);
len = strlen(str);
while (i < len) {
str[i] = toupper(str[i]);
i++;
}
printf("转换后的字符串为: %s", str);
return 0;
}
```
阅读全文
相关推荐
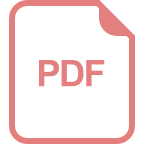
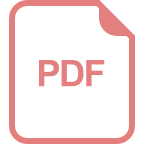
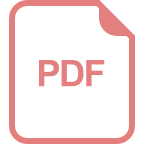












