c语言将字符串str中的小写字母改为对应的大写字母
时间: 2024-10-24 08:16:38 浏览: 75
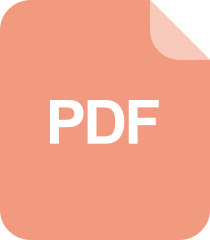
用shell脚本和c语言将大写字母转成小写的代码
在C语言中,可以使用标准库函数`toupper()`和`tolower()`来转换字符的大小写,但是这两个函数只对单个字符有效。如果你想要将整个字符串中的所有小写字母转换成大写字母,可以遍历字符串并逐个应用`toupper()`。以下是一个简单的示例:
```c
#include <ctype.h> // 包含 toupper 函数
#include <stdio.h>
#include <string.h>
void to_upper_case(char *str) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (islower(str[i])) { // 检查字符是否为小写字母
str[i] = toupper(str[i]); // 转换为大写
}
}
}
int main() {
char str[] = "Hello, World!";
to_upper_case(str);
printf("转换后的字符串: %s\n", str);
return 0;
}
```
在这个例子中,`to_upper_case`函数接收一个指向字符串的指针,并通过`for`循环检查每个字符。如果是小写字母,就使用`toupper()`将其转换为大写。
阅读全文
相关推荐
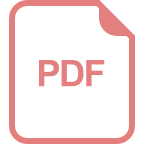
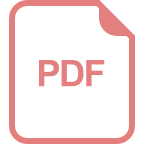









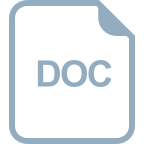





