c语言中字符串str中的小写字母全部转换成大写字母,大写字母全部转换为小写字母,其它字符不变
时间: 2024-09-25 22:02:18 浏览: 38
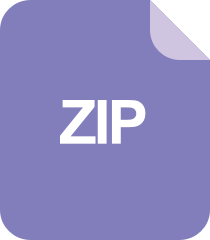
统计字符串中数字,小写字母,大写字母,空白字符和其他字符的个数
在C语言中,你可以使用标准库函数`toupper()`和`tolower()`来进行大小写的转换。如果你有一个字符串`str`,首先需要遍历整个字符串。对于每个字符,检查它是否是字母(通过`isalpha()`函数),如果是字母,则分别使用`toupper()`或`tolower()`进行转换。下面是一个简单的示例:
```c
#include <ctype.h>
#include <string.h>
void swap_case(char *str) {
for (int i = 0; str[i] != '\0'; i++) { // 遍历直到字符串结束
if (isalpha(str[i])) { // 判断字符是否为字母
if (islower(str[i])) { // 如果是小写字母,转大写
str[i] = toupper(str[i]);
} else if (isupper(str[i])) { // 如果是大写字母,转小写
str[i] = tolower(str[i]);
}
}
}
}
```
然后你可以像这样调用这个函数:
```c
char string[] = "Hello, World!";
swap_case(string);
printf("%s", string); // 输出 "hELLO, wORLD!"
```
阅读全文
相关推荐
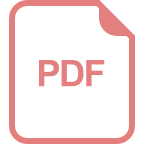
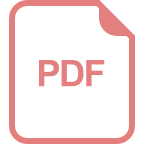















