c语言一个字符串,将此字符串中的小写字母转换为大写字母,其它字符保持不变
时间: 2024-11-27 09:20:44 浏览: 1
在C语言中,你可以使用标准库函数`toupper()`和`tolower()`来进行大小写的转换。但是需要注意的是,这两个函数只适用于ASCII字符集,对于非ASCII字符可能会有局限。下面是一个简单的例子,展示了如何遍历字符串并逐个转换小写字母:
```c
#include <ctype.h> // 包含 toupper() 和 tolower() 函数
void convert_to_upper(char* str) {
int i = 0;
while (str[i] != '\0') { // 遍历直到字符串结束
if (islower(str[i])) { // 判断字符是否为小写字母
str[i] = toupper(str[i]); // 如果是,转换为大写
}
i++; // 移动到下一个字符
}
}
```
调用这个函数,例如:
```c
int main() {
char my_string[] = "Hello, world!";
convert_to_upper(my_string);
printf("%s\n", my_string); // 输出: HELLO, WORLD!
return 0;
}
```
相关问题
c语言一个字符串,将此字符串中的小写字母转换为大写字母,其它字符保持不变 用scanf函数
在C语言中,如果你想将输入的字符串中的小写字母转换为大写字母而保留其他字符不变,可以使用标准库中的`ctype.h`头文件中的`toupper()`函数配合循环结构。下面是一个简单的示例,展示了如何使用`scanf`函数读取用户输入的字符串并逐个字符转换:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100]; // 假设字符串长度不超过99个字符
printf("请输入一个字符串: ");
// 使用scanf读取用户输入
if (scanf("%s", str) == 1) { // 检查是否成功读取了一个字符串
int len = strlen(str); // 获取字符串长度
for (int i = 0; i < len; i++) {
// 如果当前字符是小写字母,转换为大写
if (islower(str[i])) {
str[i] = toupper(str[i]);
}
}
printf("转换后的字符串: %s\n", str);
} else {
printf("输入错误!\n");
}
return 0;
}
```
c语言,将字符串中的小写字母转换为对应的大写字母 其他字符不变
可以使用C语言中的字符数组和循环结构来实现将字符串中的小写字母转换为对应的大写字母,其他字符不变。可以使用标准库函数toupper()将小写字母转换为大写字母。
下面是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i = 0;
printf("请输入一个字符串:");
scanf("%s", str);
while (str[i]) {
if (islower(str[i])) { // 判断是否为小写字母
str[i] = toupper(str[i]); // 将小写字母转换为大写字母
}
i++;
}
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
运行示例:
```
请输入一个字符串:hello world
转换后的字符串为:HELLO WORLD
```
阅读全文
相关推荐
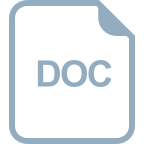
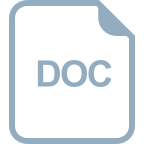
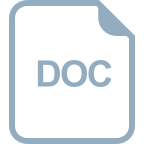












