用JS实现:题目描述:简易ATM 里面现存有 100 块钱。 如果存钱,就用输入钱数加上先存的钱数, 之后弹出显示余额提示框 如果取钱,就减去取的钱数,之后弹出显示余额提示框 如果显示余额,就输出余额 如果退出,弹出退出信息提示框 如果输入错误选项,弹出错误提示框
时间: 2024-09-17 12:03:22 浏览: 36
在JavaScript中实现一个简易的ATM模拟程序,可以使用对象和函数来表示状态和交互逻辑。下面是一个简单的例子:
```javascript
// 定义ATM对象
const atm = {
balance: 100, // 初始余额
operations: {
deposit: function(amount) {
if (amount > 0) {
this.balance += amount;
alert('存款成功,当前余额:' + this.balance);
} else {
alert('请输入正数作为存款金额');
}
},
withdraw: function(amount) {
if (amount <= this.balance) {
this.balance -= amount;
alert('取款成功,当前余额:' + this.balance);
} else {
alert('余额不足,无法完成取款');
}
},
checkBalance: function() {
alert('当前余额:' + this.balance);
},
exit: function() {
alert('已退出ATM系统');
},
inputError: function(message) {
alert(message);
}
}
};
// 模拟用户操作
function operate(option) {
switch (option) {
case 'deposit':
atm.operations.deposit(prompt('请输入存款金额:'));
break;
case 'withdraw':
atm.operations.withdraw(prompt('请输入取款金额:'));
break;
case 'checkBalance':
atm.operations.checkBalance();
break;
case 'exit':
atm.operations.exit();
break;
default:
atm.operations.inputError('无效的操作选项,请重新选择!');
}
}
// 开始循环接收用户输入并处理操作
while (true) {
const userInput = prompt('请选择操作:deposit(存款), withdraw(取款), checkBalance(查看余额), exit(退出)');
operate(userInput);
}
```
在这个示例中,我们首先创建了一个ATM对象,包含属性balance(余额)和一系列处理各种操作的方法。然后定义了一个`operate`函数用于根据用户输入调用相应的方法。通过一个无限循环,不断接收用户输入并处理操作,直到用户选择退出。
阅读全文
相关推荐
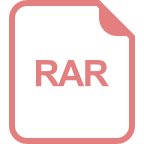
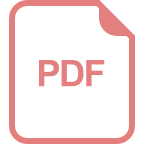
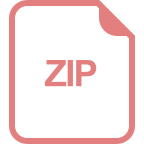
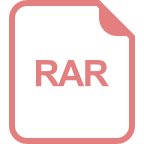
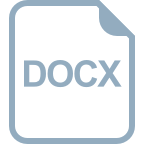
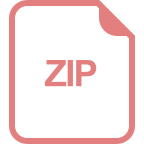
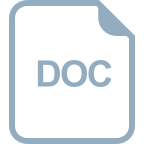
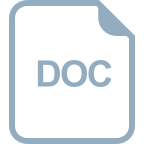
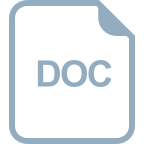






