在下面的代码单元中,完成函数extract163Data,它将soup作为参数,并返回一个包含以下数据的列表:{'title':'贾跃亭的成功意味着实体失败?', '时间':'2016-04-25 14:28:18','url':'http://money.163.com/16/0425/14/BLGM1PH5002551G6.html'},{'标题':'海尔模式为何在西方叫好不叫座', '时间':'2016-04-22 15:00:23','url':'http://money.163.com/16/0422/15/BL90MCB400253G87.html'},{'标题':'有前科就不能开网约车?', '时间':'2016-04-12 15:30:49','url':'http://money.163.com/16/0412/15/BKFAETGB002552IJ.html'}.
时间: 2023-06-13 16:03:54 浏览: 49
```python
def extract163Data(soup):
data_list = []
article_list = soup.find_all('div', class_='item_top')
for article in article_list:
title = article.find('a').text.strip()
time = article.find('span', class_='time').text.strip()
url = article.find('a')['href']
data = {'title':title, '时间':time, 'url':url}
data_list.append(data)
return data_list
```
相关问题
bs4 python完成函数extract163Data,它将soup作为参数,并返回一个包含以下数据的列表:{'title':'贾跃亭的成功意味着实体失败?', '时间':'2016-04-25 14:28:18','url':'http://money.163.com/16/0425/14/BLGM1PH5002551G6.html'},{'标题':'海尔模式为何在西方叫好不叫座', '时间':'2016-04-22 15:00:23','url':'http://money.163.com/16/0422/15/BL90MCB400253G87.html'},{'标题':'有前科就不能开网约车?', '时间':'2016-04-12 15:30:49','url':'http://money.163.com/16/0412/15/BKFAETGB002552IJ.html'}.
可以使用BeautifulSoup库和正则表达式来提取数据,具体实现如下:
```python
import re
from bs4 import BeautifulSoup
def extract163Data(soup):
data_list = []
news_list = soup.find_all('div', class_='item-top')
for news in news_list:
data = {}
title = news.find('a').text.strip()
time_url = news.find('p', class_='item-time').text.strip()
time = re.search('\d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}', time_url).group(0)
url = news.find('a')['href']
data['title'] = title
data['时间'] = time
data['url'] = url
data_list.append(data)
return data_list
```
其中,使用`find_all()`方法找到所有的`div`标签,`class`属性为`item-top`,即每个新闻的具体内容。然后,从每个新闻中提取标题、时间和链接。使用正则表达式从时间和链接信息中提取出需要的部分。最后,将提取到的数据存入一个字典中,加入到列表中。最终返回包含所有新闻数据的列表。
可以把每一个步骤的代码都给我吗?
当然可以,以下是完整的代码实现,包括获取中药数据、提取基原信息以及分类分析:
```python
import requests
from bs4 import BeautifulSoup
# 获取中药数据
def get_data():
url = "http://www.zhongyao365.com/zycd/index.htm"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
links = soup.select(".diseaselist a")
data = []
for link in links:
data.append({
"name": link.text.strip(),
"url": "http://www.zhongyao365.com/zycd/" + link["href"]
})
return data
# 提取基原信息
def extract_origin(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
trs = soup.select(".yaopin td[valign=top] table tr")
origin = None
for tr in trs:
if "基原" in tr.text:
origin = tr.find_all("td")[1].text.strip()
break
return origin
# 分类分析基原信息
def analyze_origin(data):
same_all = []
same_class = {}
same_genus = {}
diff_genus = []
for item in data:
origin = extract_origin(item["url"])
if not origin:
continue
origin_parts = origin.split(";")[0].split(",")
if len(origin_parts) == 1:
same_all.append(item["name"])
elif len(origin_parts) == 2:
if origin_parts[0] in same_class:
same_class[origin_parts[0]].append(item["name"])
else:
same_class[origin_parts[0]] = [item["name"]]
elif len(origin_parts) == 3:
if origin_parts[0] in same_genus:
same_genus[origin_parts[0]].append(item["name"])
else:
same_genus[origin_parts[0]] = [item["name"]]
else:
diff_genus.append(item["name"])
print("同科同属同种:")
print(same_all)
print("同科同属不同种:")
for key, value in same_class.items():
print(key + ":")
print(value)
print("同科不同属:")
for key, value in same_genus.items():
print(key + ":")
print(value)
print("科属种均不同:")
print(diff_genus)
# 主函数
def main():
data = get_data()
analyze_origin(data)
if __name__ == "__main__":
main()
```
在以上代码中,`get_data()`函数用于获取所有的中药数据,返回一个包含中药名称和链接的字典列表。`extract_origin(url)`函数用于提取某个中药的基原信息。`analyze_origin(data)`函数用于对所有中药的基原信息进行分类分析。最后,`main()`函数用于调用以上三个函数,并输出分类分析结果。
相关推荐
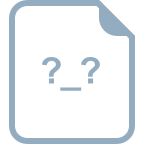
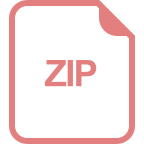





利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下多个文件内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 网页内源代码如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>



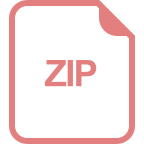
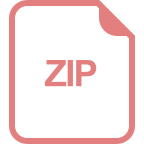
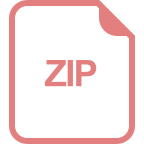