使用C语言:1.表达式求值问题,应用“栈”编写一个计算器,要求: a)支持整数(长整型范围内)四则混合运算; b)输入应是中缀表达式,对不合法的输入应有提示; c)能够输出正确计算结果; d)示例如输入5(10-3)/7,输出结果5
时间: 2024-02-11 15:09:53 浏览: 70
好的,这是一个经典的表达式求值问题,下面是使用C语言编写的计算器程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_EXPR_LEN 100
/* 定义栈的结构体 */
typedef struct {
int top; // 栈顶指针
int data[MAX_EXPR_LEN]; // 栈中存储的数据
} Stack;
/* 初始化栈 */
void stack_init(Stack *s) {
s->top = -1;
}
/* 判断栈是否为空 */
int stack_empty(Stack *s) {
return s->top == -1;
}
/* 判断栈是否已满 */
int stack_full(Stack *s) {
return s->top == MAX_EXPR_LEN - 1;
}
/* 入栈操作 */
void stack_push(Stack *s, int x) {
if (stack_full(s)) {
printf("Error: Stack is full!\n");
exit(1);
}
s->data[++s->top] = x;
}
/* 出栈操作 */
int stack_pop(Stack *s) {
if (stack_empty(s)) {
printf("Error: Stack is empty!\n");
exit(1);
}
return s->data[s->top--];
}
/* 获取栈顶元素 */
int stack_top(Stack *s) {
if (stack_empty(s)) {
printf("Error: Stack is empty!\n");
exit(1);
}
return s->data[s->top];
}
/* 判断是否为操作符 */
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
/* 获取运算符的优先级 */
int precedence(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
/* 计算两个数的结果 */
int calculate(int a, int b, char op) {
int result = 0;
switch (op) {
case '+':
result = a + b;
break;
case '-':
result = a - b;
break;
case '*':
result = a * b;
break;
case '/':
result = a / b;
break;
}
return result;
}
/* 中缀表达式转后缀表达式 */
void infix_to_postfix(char *infix, char *postfix) {
Stack s;
stack_init(&s);
int i = 0, j = 0;
while (infix[i] != '\0') {
if (isdigit(infix[i])) {
postfix[j++] = infix[i++];
while (isdigit(infix[i])) {
postfix[j++] = infix[i++];
}
postfix[j++] = ' ';
} else if (is_operator(infix[i])) {
while (!stack_empty(&s) && precedence(infix[i]) <= precedence(stack_top(&s))) {
postfix[j++] = stack_pop(&s);
postfix[j++] = ' ';
}
stack_push(&s, infix[i++]);
} else if (infix[i] == '(') {
stack_push(&s, infix[i++]);
} else if (infix[i] == ')') {
while (stack_top(&s) != '(') {
postfix[j++] = stack_pop(&s);
postfix[j++] = ' ';
}
stack_pop(&s);
i++;
} else {
printf("Error: Invalid input!\n");
exit(1);
}
}
while (!stack_empty(&s)) {
postfix[j++] = stack_pop(&s);
postfix[j++] = ' ';
}
postfix[j] = '\0';
}
/* 后缀表达式求值 */
int evaluate_postfix(char *postfix) {
Stack s;
stack_init(&s);
int i = 0;
while (postfix[i] != '\0') {
if (isdigit(postfix[i])) {
int num = 0;
while (isdigit(postfix[i])) {
num = num * 10 + postfix[i] - '0';
i++;
}
stack_push(&s, num);
} else if (postfix[i] == '+' || postfix[i] == '-' || postfix[i] == '*' || postfix[i] == '/') {
int b = stack_pop(&s);
int a = stack_pop(&s);
int result = calculate(a, b, postfix[i]);
stack_push(&s, result);
i++;
} else if (postfix[i] == ' ') {
i++;
} else {
printf("Error: Invalid input!\n");
exit(1);
}
}
return stack_pop(&s);
}
/* 主函数 */
int main() {
char infix[MAX_EXPR_LEN], postfix[MAX_EXPR_LEN];
printf("Enter an infix expression: ");
fgets(infix, MAX_EXPR_LEN, stdin);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
printf("Result: %d\n", evaluate_postfix(postfix));
return 0;
}
```
这个程序中使用了一个栈来辅助完成表达式求值的过程。首先将中缀表达式转换成后缀表达式,然后再对后缀表达式进行求值。其中,栈的结构体定义了栈顶指针和存储数据的数组,同时定义了栈的各种操作函数,包括初始化、判断是否为空、判断是否已满、入栈、出栈、获取栈顶元素等。在中缀表达式转换成后缀表达式的过程中,使用了一个循环来遍历中缀表达式中的每一个字符,根据字符的类型,分别进行相应的操作,包括将数字加入后缀表达式、将操作符入栈、将左括号入栈、将右括号出栈等。在后缀表达式求值的过程中,同样使用了一个循环来遍历后缀表达式中的每一个字符,根据字符的类型,分别进行相应的操作,包括将数字入栈、将操作符出栈并计算结果、跳过空格等。最后,输出计算结果即可。
阅读全文
相关推荐
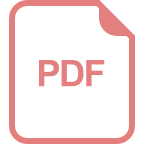
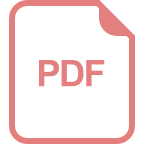
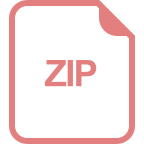








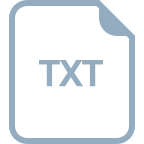
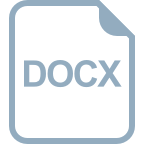
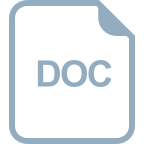
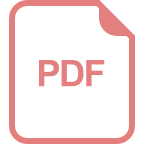
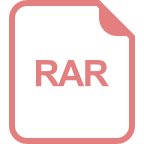
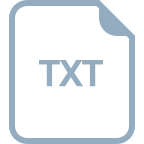
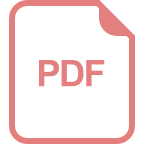
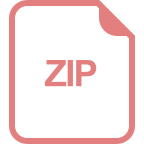