c#利用循环计算n!的值 n!=1*23*….*
时间: 2023-07-23 19:05:19 浏览: 160
可以使用 for 循环来计算 n! 的值,每次将当前的数字乘以上一个数字,一直乘到 n。
下面是一个使用 for 循环计算 n! 的示例代码:
```csharp
int n = 5;
int factorial = 1;
for (int i = 1; i <= n; i++)
{
factorial *= i;
}
Console.WriteLine("{0}! = {1}", n, factorial);
```
输出结果为:
```
5! = 120
```
这个代码中,我们先定义了要计算的数字 n 和结果变量 factorial,然后使用 for 循环从 1 到 n 遍历,每次将当前的数字乘以上一个数字,并将结果赋值给 factorial。最后输出计算结果。
相关问题
c#利用循环计算n!的值 n!=1*2*3*….*n
可以使用一个循环来计算 n! 的值。具体步骤如下:
1. 定义一个变量 result,初始值为 1。
2. 使用一个循环从 1 循环到 n,每次将 result 乘以当前循环变量的值,即 result *= i。
3. 循环结束后,result 的值即为 n! 的值。
下面是相应的 C# 代码实现:
```csharp
int n = 5; // 代表要计算 5!
int result = 1;
for (int i = 1; i <= n; i++)
{
result *= i;
}
Console.WriteLine($"{n}! = {result}");
```
输出结果为:5! = 120。
编写一个c#程序:输入整数n (n大于等于0),分别利用for循环、while 循环、do...while循环求n!。↓
在C#中,计算阶乘(n!)通常涉及到一个循环结构,可以使用`for`、`while`和`do...while`三种方式进行。以下是分别使用这三种循环结构计算阶乘的示例程序:
### 1. 使用for循环
```csharp
using System;
class Program
{
static void Main()
{
Console.Write("请输入一个非负整数:");
int n = Convert.ToInt32(Console.ReadLine());
long factorial = 1; // 初始化阶乘为1
for (int i = 1; i <= n; i++)
{
factorial *= i;
}
Console.WriteLine($"n! = {factorial}");
}
}
```
### 2. 使用while循环
```csharp
using System;
class Program
{
static void Main()
{
Console.Write("请输入一个非负整数:");
int n = Convert.ToInt32(Console.ReadLine());
long factorial = 1;
int i = 1;
while (i <= n)
{
factorial *= i;
i++;
}
Console.WriteLine($"n! = {factorial}");
}
}
```
### 3. 使用do...while循环
```csharp
using System;
class Program
{
static void Main()
{
Console.Write("请输入一个非负整数:");
int n = Convert.ToInt32(Console.ReadLine());
long factorial = 1;
int i = 1;
do
{
factorial *= i;
i++;
} while (i <= n);
Console.WriteLine($"n! = {factorial}");
}
}
```
在这三个例子中,都会检查给定的`n`是否大于0,然后计算从1到`n`的所有整数相乘的结果。
阅读全文
相关推荐
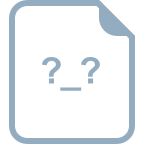
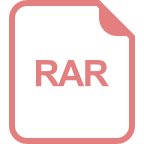
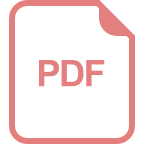
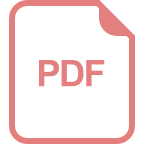
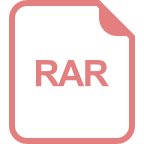
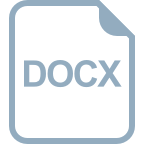








