使用perl写一个通过ak与sk去认证调用华为云刷新cdn缓存预热的api接口
时间: 2024-05-06 07:19:36 浏览: 144
以下是使用Perl编写的代码示例,用于调用华为云刷新CDN缓存预热API接口:
```
#!/usr/bin/perl
use strict;
use warnings;
use LWP::UserAgent;
use JSON qw(encode_json);
# Set the AK and SK
my $ak = '<YOUR_AK>';
my $sk = '<YOUR_SK>';
# Set the API endpoint and request URL
my $endpoint = 'https://cdn.myhuaweicloud.com';
my $request_url = '/v1.0/refreshtasks';
# Set the request body parameters
my $request_body = {
'tasks' => [
{
'type' => 'flush',
'urls' => [
'http://example.com',
'https://example.com'
]
},
{
'type' => 'preheating',
'urls' => [
'http://example.com',
'https://example.com'
],
'urls_type' => 'file',
'area' => 'mainland',
'refresh_type' => 'url'
}
]
};
# Encode the request body parameters as JSON
my $json_request_body = encode_json($request_body);
# Generate the authorization header
my $date = `date -u +%a, %d %b %Y %H:%M:%S GMT`;
chomp($date);
my $auth_header = 'HWS ' . $ak . ':' . hmac_sha1_hex($date, $sk);
# Create a new LWP::UserAgent object
my $ua = LWP::UserAgent->new;
# Set the request headers
my $request_headers = [
'Content-Type' => 'application/json',
'X-Auth-Date' => $date,
'Authorization' => $auth_header
];
# Send the API request
my $response = $ua->post($endpoint . $request_url, content => $json_request_body, headers => $request_headers);
# Check for errors
if ($response->is_success) {
print "API request successful!\n";
print "Response:\n" . $response->content . "\n";
} else {
print "API request failed!\n";
print "Response:\n" . $response->status_line . "\n";
}
```
注意:在此示例中,我们使用了HMAC-SHA1算法来生成授权头。您需要安装Crypt::Digest::SHA1模块来使用此算法。您还需要将YOUR_AK和YOUR_SK替换为您自己的华为云AK和SK。
阅读全文
相关推荐
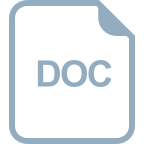
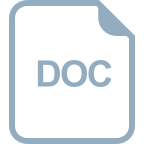
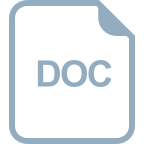



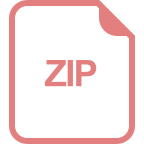
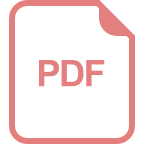
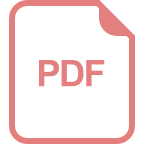
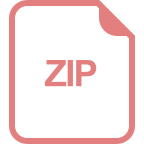
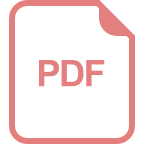
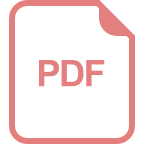
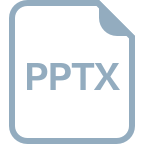
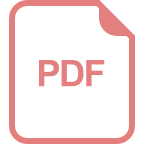
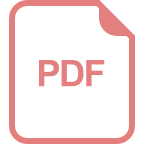
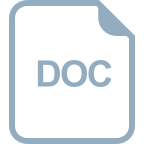


