求每个系的学生的平均年龄,并把结果存入数据库中
时间: 2023-06-14 22:06:42 浏览: 355
这个任务需要获取每个系的学生列表,然后计算平均年龄并将结果存入数据库。具体步骤如下:
1. 连接数据库并查询每个系的学生列表。假设我们有一个名为 students 的表,其中包含以下列:id, name, age, department。我们可以使用以下 SQL 语句获取每个系的学生列表:
```
SELECT department, AVG(age) AS avg_age FROM students GROUP BY department;
```
2. 将结果存入数据库。假设我们有一个名为 department_avg_age 的表,其中包含以下列:id, department, avg_age。我们可以使用以下 SQL 语句将结果存入数据库:
```
INSERT INTO department_avg_age (department, avg_age) VALUES ('系别1', 20.5);
INSERT INTO department_avg_age (department, avg_age) VALUES ('系别2', 21.3);
INSERT INTO department_avg_age (department, avg_age) VALUES ('系别3', 22.1);
```
其中,系别1、系别2、系别3 分别是每个系的名称,20.5、21.3、22.1 分别是每个系的平均年龄。
3. 如果需要自动化这个任务,可以编写一个脚本来执行以上步骤。具体实现方式取决于所使用的编程语言和数据库。例如,使用 Python 和 MySQL 可以使用以下代码:
```python
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
mycursor = mydb.cursor()
mycursor.execute("""
SELECT department, AVG(age) AS avg_age FROM students GROUP BY department;
""")
results = mycursor.fetchall()
for result in results:
department = result[0]
avg_age = result[1]
sql = "INSERT INTO department_avg_age (department, avg_age) VALUES (%s, %s)"
val = (department, avg_age)
mycursor.execute(sql, val)
mydb.commit()
print(mycursor.rowcount, "records inserted.")
```
这段代码连接到名为 yourdatabase 的数据库,执行 SQL 查询语句获取每个系的平均年龄,并将结果插入到名为 department_avg_age 的表中。注意,这段代码只是示例,实际应用中需要根据具体情况进行修改。
阅读全文
相关推荐
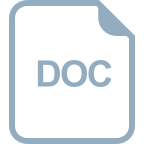
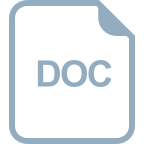
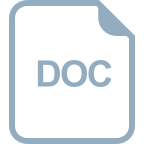

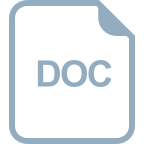
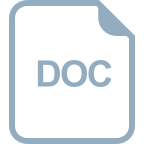
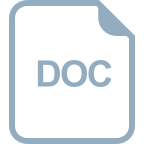
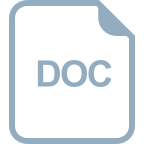
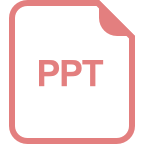
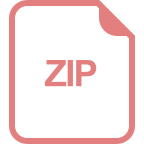
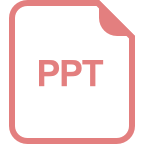
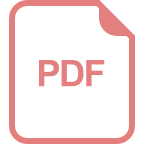
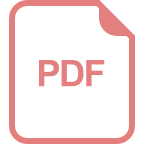
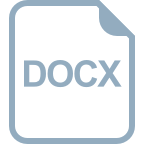
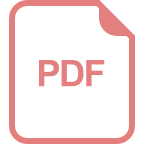
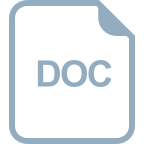
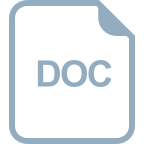
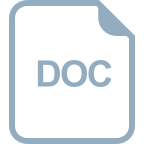
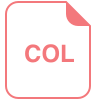