编写一段共性缓存RTL代码
时间: 2024-03-02 09:29:03 浏览: 80
以下是一段共性缓存RTL代码的示例:
```verilog
module cache (
input wire clk,
input wire rst_n,
input wire [31:0] addr_in,
input wire [31:0] data_in,
input wire we_in,
output wire [31:0] data_out,
input wire [31:0] addr_out
);
// Parameters
parameter CACHE_SIZE = 1024; // Cache size in bytes
parameter CACHE_LINE_SIZE = 32; // Cache line size in bytes
parameter CACHE_ASSOCIATIVITY = 4; // Cache associativity
parameter CACHE_NUM_SETS = CACHE_SIZE / (CACHE_LINE_SIZE * CACHE_ASSOCIATIVITY); // Number of sets in cache
parameter CACHE_INDEX_WIDTH = $clog2(CACHE_NUM_SETS); // Width of index field
parameter CACHE_TAG_WIDTH = 32 - CACHE_INDEX_WIDTH - $clog2(CACHE_LINE_SIZE); // Width of tag field
// Internal signals
wire [CACHE_TAG_WIDTH-1:0] tag_in;
wire [CACHE_INDEX_WIDTH-1:0] index_in;
wire [CACHE_TAG_WIDTH-1:0] tag_out;
wire [CACHE_INDEX_WIDTH-1:0] index_out;
wire [CACHE_LINE_SIZE-1:0] data_out_internal;
reg [CACHE_TAG_WIDTH-1:0] tag_array [0:CACHE_NUM_SETS-1][0:CACHE_ASSOCIATIVITY-1];
reg [CACHE_LINE_SIZE-1:0] data_array [0:CACHE_NUM_SETS-1][0:CACHE_ASSOCIATIVITY-1];
reg [CACHE_ASSOCIATIVITY-1:0] valid_array [0:CACHE_NUM_SETS-1];
wire [CACHE_ASSOCIATIVITY-1:0] hit_index;
wire hit;
// Extract tag and index from address
assign tag_in = addr_in[CACHE_TAG_WIDTH+CACHE_INDEX_WIDTH-1:CACHE_INDEX_WIDTH];
assign index_in = addr_in[CACHE_TAG_WIDTH+CACHE_INDEX_WIDTH-1:CACHE_TAG_WIDTH];
// Check for hit in cache
assign hit = valid_array[index_out][hit_index] && (tag_out == tag_in);
// Extract tag and index from output address
assign tag_out = addr_out[CACHE_TAG_WIDTH+CACHE_INDEX_WIDTH-1:CACHE_INDEX_WIDTH];
assign index_out = addr_out[CACHE_TAG_WIDTH+CACHE_INDEX_WIDTH-1:CACHE_TAG_WIDTH];
// Write to cache
always @(posedge clk) begin
if (!rst_n) begin
// Reset cache
for (int i=0; i<CACHE_NUM_SETS; i=i+1) begin
for (int j=0; j<CACHE_ASSOCIATIVITY; j=j+1) begin
tag_array[i][j] <= 0;
data_array[i][j] <= 0;
end
valid_array[i] <= 0;
end
end else if (we_in) begin
// Write data to cache
if (hit) begin
// Update existing line
data_array[index_out][hit_index] <= data_in;
end else begin
// Replace existing line or select empty line
int replace_index = CACHE_ASSOCIATIVITY;
for (int i=0; i<CACHE_ASSOCIATIVITY; i=i+1) begin
if (!valid_array[index_out][i]) begin
// Found empty line
replace_index = i;
break;
end
end
if (replace_index == CACHE_ASSOCIATIVITY) begin
// No empty line found, replace LRU line
replace_index = 0;
for (int i=1; i<CACHE_ASSOCIATIVITY; i=i+1) begin
if (valid_array[index_out][i] && (valid_array[index_out][i-1] == 0 || $time - valid_array[index_out][i] < $time - valid_array[index_out][i-1])) begin
replace_index = i;
end
end
end
tag_array[index_out][replace_index] <= tag_in;
data_array[index_out][replace_index] <= data_in;
valid_array[index_out][replace_index] <= $time;
end
end
end
// Read from cache
assign data_out_internal = data_array[index_out][hit_index];
assign data_out = hit ? data_out_internal : 32'h00000000;
// Find hit index
assign hit_index = 0;
for (int i=0; i<CACHE_ASSOCIATIVITY; i=i+1) begin
if (valid_array[index_out][i] && tag_array[index_out][i] == tag_out) begin
hit_index = i;
end
end
endmodule
```
此模块实现了一个具有指定大小、行大小和关联度的缓存。输入地址和数据用于读写缓存,而输出数据则根据是否命中来决定。此外,此模块还包含了一些内部信号和参数,例如标记、索引、缓存数组、有效位和更新策略。这个实现仅仅是一个示例,实际实现需要视具体需求而定。
阅读全文
相关推荐
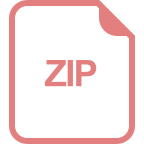
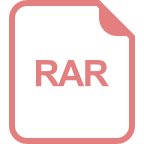
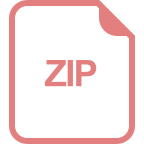
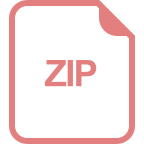
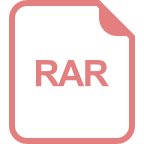
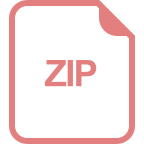
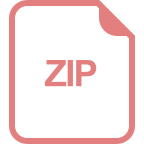
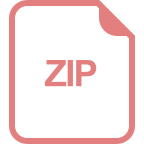
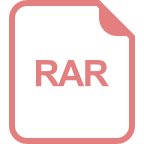
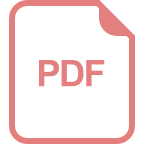
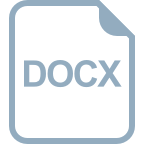
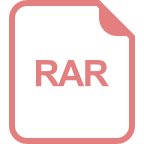
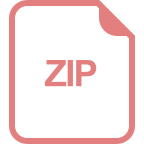
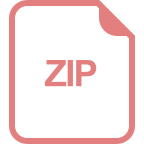