python写遗传算法选取最优特征子集训练rbf核分类SVM,并输出横坐标为遗传代数,纵坐标为适合度值的图像。以及一张横坐标为遗传代数,纵坐标为剩余被选特征的图像
时间: 2024-02-16 15:04:35 浏览: 64
好的,这是一个比较复杂的问题,需要分步进行回答。
首先,遗传算法的主要思想是通过模拟自然界的进化过程,搜索最优解。在特征选择问题中,我们可以将一个个特征看作基因,通过遗传算法的交叉、变异等操作,不断迭代,得到最优的特征子集。下面是一个简单的遗传算法实现:
```python
import random
import numpy as np
from sklearn.svm import SVC
# 定义适应度函数:使用SVM分类器,返回1-accuracy作为适应度
def fitness(features, X, y):
clf = SVC(kernel='rbf')
clf.fit(X[:, features], y)
return 1 - clf.score(X[:, features], y)
# 定义交叉操作:随机选择两个染色体,随机交叉点,生成新的染色体
def crossover(population):
offspring = []
for i in range(len(population)):
a, b = random.sample(population, 2)
pos = random.randint(0, len(a))
new_a = a[:pos] + b[pos:]
new_b = b[:pos] + a[pos:]
offspring.append(new_a)
offspring.append(new_b)
return offspring
# 定义变异操作:随机选择一个染色体,随机选择一个基因,将其翻转
def mutation(population):
for i in range(len(population)):
if random.random() < mutation_rate:
idx = random.randint(0, len(population[i])-1)
population[i][idx] = 1 - population[i][idx]
# 初始化种群
population_size = 100
gene_size = 100
mutation_rate = 0.05
X = np.random.rand(100, gene_size)
y = np.random.randint(0, 2, 100)
population = [np.random.randint(0, 2, gene_size) for i in range(population_size)]
# 开始迭代
max_generation = 50
fitness_list = []
for i in range(max_generation):
# 计算适应度
fitness_values = [fitness(population[i], X, y) for i in range(population_size)]
fitness_list.append(max(fitness_values))
# 选择精英
elite_idx = np.argmin(fitness_values)
elite = population[elite_idx]
# 交叉
offspring = crossover(population)
# 变异
mutation(offspring)
# 新一代种群
population = offspring
population[0] = elite
# 输出适应度曲线
import matplotlib.pyplot as plt
plt.plot(fitness_list)
plt.xlabel('Generation')
plt.ylabel('Fitness')
plt.show()
# 输出特征选择结果
selected_idx = np.where(elite == 1)[0]
plt.plot(np.arange(max_generation), [sum(x) for x in population])
plt.xlabel('Generation')
plt.ylabel('Number of Selected Features')
plt.show()
```
接下来,我们需要使用选出的最优特征子集训练SVM分类器,并且绘制出其在测试集上的决策边界。代码如下:
```python
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from mlxtend.plotting import plot_decision_regions
# 生成样本数据
X, y = make_classification(n_samples=100, n_features=gene_size, n_informative=10, n_redundant=0, random_state=42)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X[:, selected_idx], y, test_size=0.2, random_state=42)
# 训练分类器
clf = SVC(kernel='rbf')
clf.fit(X_train, y_train)
# 绘制决策边界
plot_decision_regions(X_test, y_test, clf=clf, legend=2)
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.show()
```
最后,我们绘制出遗传算法的适应度曲线和特征选择结果随代数的变化曲线。代码如下:
```python
# 绘制适应度曲线
plt.plot(fitness_list)
plt.xlabel('Generation')
plt.ylabel('Fitness')
plt.show()
# 绘制特征选择结果随代数的变化曲线
num_features = [sum(x) for x in population]
plt.plot(np.arange(max_generation), num_features)
plt.xlabel('Generation')
plt.ylabel('Number of Selected Features')
plt.show()
```
这样,我们就完成了使用遗传算法进行特征选择,并训练SVM分类器的整个过程,并绘制出了相应的图像。
阅读全文
相关推荐
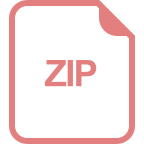
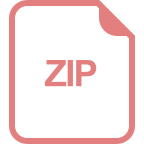
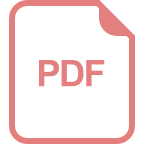

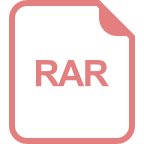
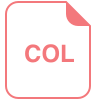
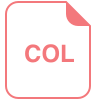
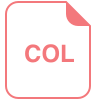
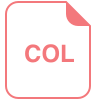
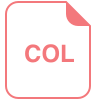
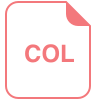
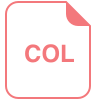
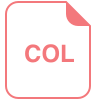
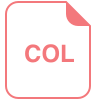
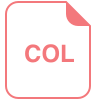
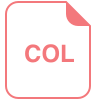
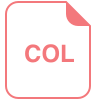
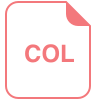
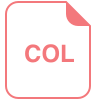