pytorch 张量乘法
时间: 2023-10-16 15:22:11 浏览: 57
PyTorch中可以使用`torch.matmul()`函数进行张量乘法。如果需要进行逐元素乘法,则可以使用`torch.mul()`函数。
下面是一个示例代码,展示如何使用`torch.matmul()`进行张量乘法:
``` python
import torch
# 创建两个张量
x = torch.randn(2, 3)
y = torch.randn(3, 4)
# 进行张量乘法
z = torch.matmul(x, y)
# 打印结果
print(z)
```
输出:
```
tensor([[ 0.5614, 0.2957, 0.8999, -0.0384],
[-0.6807, -1.6377, 0.8762, -0.4621]])
```
如果需要进行逐元素乘法,可以使用`torch.mul()`函数,示例如下:
``` python
import torch
# 创建两个张量
x = torch.randn(2, 3)
y = torch.randn(2, 3)
# 进行逐元素乘法
z = torch.mul(x, y)
# 打印结果
print(z)
```
输出:
```
tensor([[-0.3223, 0.6475, 0.3215],
[-0.0222, 0.1238, 0.0279]])
```
相关问题
pytorch 张量
PyTorch张量是PyTorch框架中的核心数据结构之一。它类似于NumPy的多维数组,但提供了GPU加速和自动求导等功能。
在PyTorch中,张量可以是标量(0维张量)、向量(1维张量)、矩阵(2维张量)或更高维的数组。可以使用torch.Tensor类创建张量对象。
以下是一个创建和操作PyTorch张量的示例:
```python
import torch
# 创建一个空的3x3浮点型张量
x = torch.empty(3, 3)
print(x)
# 创建一个随机初始化的3x3浮点型张量
y = torch.rand(3, 3)
print(y)
# 创建一个全零的整型张量
z = torch.zeros(2, 2, dtype=torch.int)
print(z)
# 使用数据直接创建张量
data = [1, 2, 3, 4]
a = torch.tensor(data)
print(a)
# 张量的形状
print(a.shape)
# 张量的大小
print(a.size())
# 张量的运算
b = torch.tensor([5, 6, 7, 8])
c = a + b
print(c)
```
以上代码演示了创建、形状、大小和运算等基本操作。PyTorch还提供了丰富的张量操作和数学函数,可以进行矩阵乘法、转置、索引、切片等操作。
pytorch张量是什么
PyTorch张量(Tensor)是一个多维数组,类似于Numpy数组。PyTorch张量由数据和形状(维度)组成,可以在GPU上运行加速计算,也可以进行自动微分。与Numpy数组不同的是,PyTorch张量可以在GPU上运行,而且可以使用自动微分功能来计算梯度。同时,PyTorch张量也支持各种数学操作,如加减乘除、矩阵乘法、广播等。PyTorch张量是PyTorch深度学习框架的基础数据结构之一,被广泛应用于深度学习模型的构建和训练中。
相关推荐
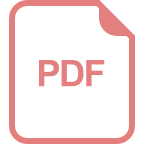
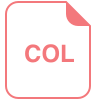
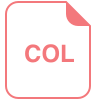
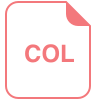
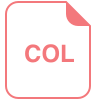
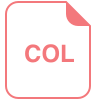







