pytorch张量操作
时间: 2023-10-19 21:19:38 浏览: 56
PyTorch中的张量操作可以分为以下几类:
1. 创建张量:
```python
import torch
# 创建一个大小为(3, 2)的张量
tensor1 = torch.tensor([[1, 2], [3, 4], [5, 6]])
print(tensor1)
# 创建一个大小为(2, 2)的全0张量
tensor2 = torch.zeros((2, 2))
print(tensor2)
# 创建一个大小为(2, 2)的全1张量
tensor3 = torch.ones((2, 2))
print(tensor3)
# 创建一个大小为(3, 3)的随机张量
tensor4 = torch.rand((3, 3))
print(tensor4)
```
2. 张量运算:
```python
# 张量加法
tensor5 = torch.tensor([[1, 2], [3, 4]])
tensor6 = torch.tensor([[5, 6], [7, 8]])
tensor7 = tensor5 + tensor6
print(tensor7)
# 张量乘法
tensor8 = torch.tensor([[1, 2], [3, 4]])
tensor9 = torch.tensor([[5, 6], [7, 8]])
tensor10 = torch.matmul(tensor8, tensor9)
print(tensor10)
# 张量求和
tensor11 = torch.tensor([[1, 2], [3, 4]])
tensor12 = torch.sum(tensor11)
print(tensor12)
# 张量转置
tensor13 = torch.tensor([[1, 2], [3, 4], [5, 6]])
tensor14 = torch.transpose(tensor13, 0, 1)
print(tensor14)
```
3. 索引和切片:
```python
# 张量索引
tensor15 = torch.tensor([[1, 2], [3, 4]])
print(tensor15[0, 1])
# 张量切片
tensor16 = torch.tensor([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(tensor16[:, 1])
```
4. 改变张量形状:
```python
# 改变张量形状
tensor17 = torch.tensor([[1, 2], [3, 4], [5, 6]])
tensor18 = tensor17.view(2, 3)
print(tensor18)
```
相关推荐
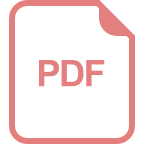
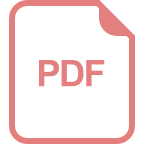















