vue 中如何获取list中的某元素
时间: 2024-05-08 22:21:39 浏览: 8
在 Vue 中,获取 list 中的某个元素可以使用数组下标的方式进行访问,例如:
```javascript
<template>
<div>
<ul>
<li v-for="(item, index) in list" :key="index">
{{ item }}
<button @click="getItem(index)">获取元素</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
list: ['元素1', '元素2', '元素3']
}
},
methods: {
getItem(index) {
console.log(this.list[index])
}
}
}
</script>
```
在上述代码中,我们通过 `v-for` 指令来遍历 `list` 数组,然后在模板中展示每个元素的值,并且为每个元素添加了一个按钮,点击按钮可以获取到对应元素的值。在 `getItem` 方法中,我们通过数组下标的方式获取到对应元素的值,并将其打印在控制台中。
相关问题
vue 删除数组中的某值
在Vue中删除数组中的某个值可以使用splice方法。首先,你需要找到要删除的值在数组中的索引位置。可以使用findIndex方法来找到该值的索引。然后,使用splice方法将该索引位置的元素从数组中删除。下面是一个示例代码:
```javascript
let arr = \[1, 2, 3, 4, 5\];
let index = arr.findIndex(item => {
if (item === 3) {
return true;
}
});
arr.splice(index, 1);
```
在上面的代码中,我们找到了值为3的元素在数组中的索引位置,并使用splice方法将其删除。最终的结果是\[1, 2, 4, 5\]。
#### 引用[.reference_title]
- *1* *2* [vue 删除数组中的某一条数据](https://blog.csdn.net/weixin_49296016/article/details/118388064)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
vue中splice
在Vue中,splice()方法可以用于替换/删除/添加数组内某一个或者几个值,该方法会改变原始数组。具体使用方法如下:
```javascript
// 在Vue组件中定义一个数组
data() {
return {
list: ['a', 'b', 'c', 'd']
}
}
// 在Vue组件中使用splice()方法
this.list.splice(1, 2, 'x', 'y') // 从索引1开始删除2个元素,并添加'x'和'y',最终数组为['a', 'x', 'y', 'd']
```
需要注意的是,Vue中的数组变异方法(如splice())会触发视图更新,因此应该尽量避免直接修改数组,而是应该使用Vue提供的变异方法来操作数组。
相关推荐
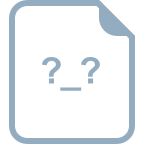












