数据结构银行窗口排队代码c++
时间: 2023-07-30 11:12:00 浏览: 95
下面是一个使用数据结构实现银行窗口排队的C++代码示例:
```c++
#include <iostream>
#include <list>
#include <string>
using namespace std;
class Customer {
public:
string name;
int serviceTime;
Customer(string n, int t) {
name = n;
serviceTime = t;
}
};
class BankQueue {
private:
list<Customer> queue;
int totalServiceTime;
public:
BankQueue() {
totalServiceTime = 0;
}
bool isEmpty() {
return queue.empty();
}
void addCustomer(Customer c) {
queue.push_back(c);
}
void serveCustomer() {
if (!isEmpty()) {
totalServiceTime += queue.front().serviceTime;
cout << "Serving customer " << queue.front().name << " for " << queue.front().serviceTime << " seconds.\n";
queue.pop_front();
} else {
cout << "No customers in the queue.\n";
}
}
int getTotalServiceTime() {
return totalServiceTime;
}
};
int main() {
BankQueue queue;
string name;
int serviceTime;
char option;
do {
cout << "Enter option (a=arrive, d=depart, q=quit): ";
cin >> option;
switch (option) {
case 'a':
cout << "Enter customer name: ";
cin >> name;
cout << "Enter service time (in seconds): ";
cin >> serviceTime;
queue.addCustomer(Customer(name, serviceTime));
cout << name << " added to the queue.\n";
break;
case 'd':
queue.serveCustomer();
break;
case 'q':
cout << "Total service time: " << queue.getTotalServiceTime() << " seconds.\n";
cout << "Quitting program.\n";
break;
default:
cout << "Invalid option.\n";
}
} while (option != 'q');
return 0;
}
```
这段代码使用一个双向链表来存储排队的顾客。当一个顾客到达时,他们的名字和需要的服务时间会被添加到队列的末尾。当一个顾客离开时,他们的名字和服务时间会从队列的前面移除,并打印一条消息告诉我们该顾客已经被服务。当我们要退出程序时,我们可以输入 'q',并打印出总服务时间。
阅读全文
相关推荐
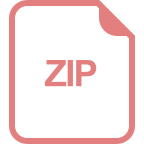
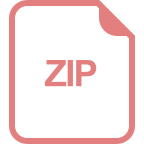

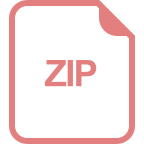
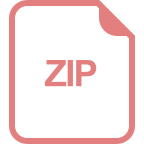
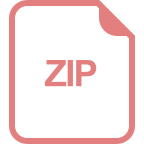
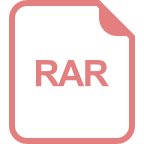
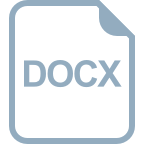
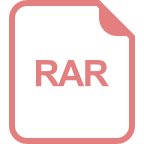
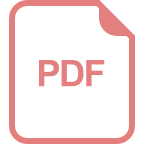
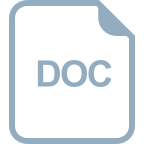
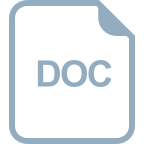
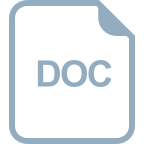
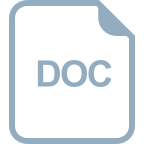

