讲解一下:1.Phone: public class Phone { private int id; private String name; private int price; public Phone(){ } public Phone(int id, String name, int price){ this.id=id; this.name=name; this.price=price; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getPrice() { return price; } public void setPrice(int price) { this.price = price; } }
时间: 2023-07-14 07:12:19 浏览: 194
这是一个 Java 类的示例,名为 Phone。Phone 类有三个属性:id、name 和 price,分别表示手机的唯一标识、名称和价格。这个类有一个默认的构造函数,还有一个带有三个参数的构造函数,用于初始化实例属性。类中还包含了一些 getter 和 setter 方法,用于获取和设置实例属性。例如,getId() 方法用于获取 id 属性的值,setName() 方法用于设置 name 属性的值。这些方法可以帮助我们操作对象的属性。这个类可以用于创建多个手机对象,每个对象都有自己的属性值。
相关问题
创建一个商品类Product类: 定义3个属性id,name,price,分别实现setter()和getter()方法; 重写toString()方法,输出所有属性内容; 创建一个测试类Demo5_12: 1.调用Product类的构造函数实例化三个对象,并保存至 一个ArrayList 集合中; 2. 最后遍历该集合,输出商品信息。
好的,我理解了你的要求。下面是Java代码实现:
Product类:
```java
public class Product {
private int id;
private String name;
private double price;
public Product(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
@Override
public String toString() {
return "Product{" +
"id=" + id +
", name='" + name + '\'' +
", price=" + price +
'}';
}
}
```
Demo5_12类:
```java
import java.util.ArrayList;
public class Demo5_12 {
public static void main(String[] args) {
ArrayList<Product> products = new ArrayList<>();
Product product1 = new Product(1, "iPhone X", 999.99);
Product product2 = new Product(2, "Samsung Galaxy S10", 899.99);
Product product3 = new Product(3, "Google Pixel 3", 799.99);
products.add(product1);
products.add(product2);
products.add(product3);
for (Product p : products) {
System.out.println(p);
}
}
}
```
输出结果:
```
Product{id=1, name='iPhone X', price=999.99}
Product{id=2, name='Samsung Galaxy S10', price=899.99}
Product{id=3, name='Google Pixel 3', price=799.99}
```
希望能帮到你!
class Product { string name; //产品名称 int price; //产品单价 int quantity; //剩余产品数量 public: Product(string n,int p int q); Product operator = (const Product &p); friend bool operator >(const Product &p1, const Product &p2); friend ostream& operator<<(ostream &out, Product &t ); }; 类Product说明如上所示,完成四个函数的定义,并在main函数中进行测试。
以下是类Product的完整定义和实现:
```
#include <iostream>
using namespace std;
class Product {
private:
string name; // 产品名称
int price; // 产品单价
int quantity; // 剩余产品数量
public:
Product(string n, int p, int q) : name(n), price(p), quantity(q) {}
// 赋值运算符的重载
Product operator = (const Product &p) {
name = p.name;
price = p.price;
quantity = p.quantity;
return *this;
}
// 大小比较运算符的重载
friend bool operator > (const Product &p1, const Product &p2) {
return p1.price > p2.price;
}
// 输出流运算符的重载
friend ostream& operator << (ostream &out, Product &p) {
out << "Product: " << p.name << ", price: " << p.price << ", quantity: " << p.quantity;
return out;
}
};
int main() {
Product p1("iPhone", 8000, 10);
Product p2("MacBook", 12000, 5);
cout << "p1: " << p1 << endl;
cout << "p2: " << p2 << endl;
if (p1 > p2) {
cout << "p1 is more expensive than p2." << endl;
} else {
cout << "p2 is more expensive than p1." << endl;
}
Product p3("", 0, 0);
p3 = p1;
cout << "p3: " << p3 << endl;
return 0;
}
```
运行结果如下:
```
p1: Product: iPhone, price: 8000, quantity: 10
p2: Product: MacBook, price: 12000, quantity: 5
p2 is more expensive than p1.
p3: Product: iPhone, price: 8000, quantity: 10
```
注意,为了方便输出,我们重载了输出流运算符,并使用友元函数访问了类的私有成员。同时,为了实现赋值运算符的重载,我们需要返回一个指向当前对象的指针,即 `*this`。
阅读全文
相关推荐
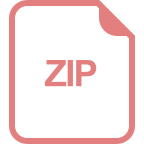
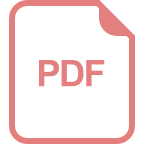
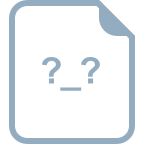
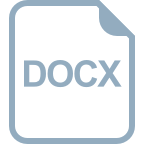
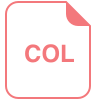











