统计字符串中某一字符串出现的次数用c++
时间: 2023-03-30 16:03:07 浏览: 115
可以使用 C 语言中的字符串函数来统计字符串中某一字符串出现的次数,例如使用 strstr 函数来查找目标字符串,然后使用循环来计数出现的次数。以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "hello world, world is beautiful";
char target[] = "world";
int count = ;
char *ptr = str;
while ((ptr = strstr(ptr, target)) != NULL) {
count++;
ptr += strlen(target);
}
printf("'%s' appears %d times in '%s'\n", target, count, str);
return ;
}
```
输出结果为:
```
'world' appears 2 times in 'hello world, world is beautiful'
```
相关问题
C++ 统计字符串中某字符出现的次数
可以循环遍历字符串中的每一个字符,然后判断是否和目标字符相等,如果相等计数器加一。这里提供一个示例代码:
```C++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
char target = 'o';
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str[i] == target) {
count++;
}
}
cout << target << " appears " << count << " times in the string." << endl;
return 0;
}
```
输出结果为:
```
o appears 2 times in the string.
```
用c++写统计某个字符在字符串中出现的次数
可以使用 C++ 的字符串(string)类和循环结构实现统计某个字符在字符串中出现的次数。具体实现如下:
```cpp
#include <iostream>
#include <string>
using namespace std;
int countChar(string str, char c) {
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str[i] == c) {
count++;
}
}
return count;
}
int main() {
string str = "Hello, World!";
char c = 'o';
int count = countChar(str, c);
cout << "The character '" << c << "' appears " << count << " times in the string." << endl;
return 0;
}
```
在上面的代码中,我们定义了一个 `countChar` 函数,它接收两个参数:一个字符串和一个字符。该函数使用循环结构遍历字符串中的每个字符,如果当前字符等于要统计的字符,就将计数器加 1。最后,函数返回计数器的值。
在 `main` 函数中,我们定义了一个字符串 `str` 和一个字符 `c`,然后调用 `countChar` 函数统计字符 `c` 在字符串 `str` 中出现的次数,并将结果输出到控制台。
相关推荐
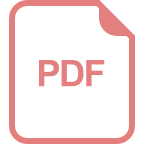
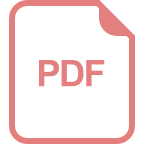












