如何在C语言中写入文件?
时间: 2024-09-06 09:01:01 浏览: 44
在C语言中,你可以使用`stdio.h`库中的文件操作函数来向文件写入内容。以下是两个主要的示例:
1. **写入文本**[^1]:
```c
#include <stdio.h>
int main() {
FILE *fp = fopen("myfile.txt", "w"); // 以写入模式打开文件
if (fp == NULL) {
printf("Error opening file!\n");
return 1;
}
// 使用fprintf()写入字符串
fprintf(fp, "Hello, world!\n");
// 使用fputs()写入另一个字符串
fputs("This is another string.\n", fp);
// 关闭文件
fclose(fp);
return 0;
}
```
2. **写入二进制数据**:
```c
int num = 42; // 要写入的数据
fwrite(&num, sizeof(int), 1, fp); // 使用fwrite写入整数
```
3. **读取并打印文本**[^2]:
```c
#include <stdio.h>
int main() {
FILE* fp = fopen("text.txt", "r"); // 以读取模式打开文件
char str[1024];
while (fscanf(fp, "%s", str) != EOF) { // 使用fscanf逐行读取
printf("%s", str);
}
fclose(fp);
return 0;
}
```
阅读全文
相关推荐
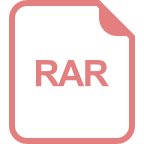
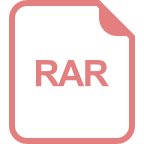
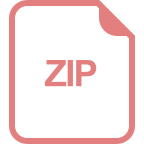
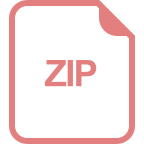
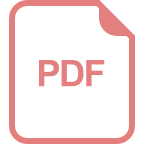
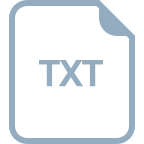
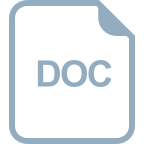
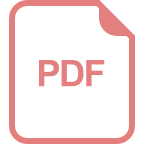
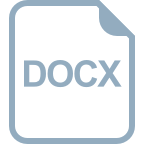
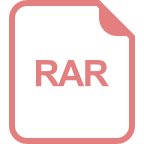
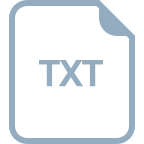
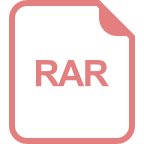
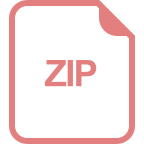
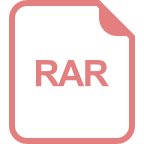
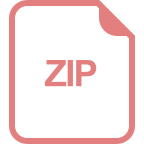
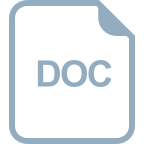
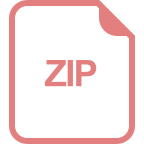