matlab 寻峰算法
时间: 2023-05-13 09:00:27 浏览: 343
寻峰算法是一种在MATLAB 中用于找到数据集中具有最大值的峰值的算法。这个算法可以用于多种不同的应用场景,比如声学分析、图像处理、化学分析等等。 寻峰算法实现的主要思路就是在数据集中搜索局部最大值点,以确定峰值的位置。一般而言,算法中首先要对数据集进行预处理,以消除噪音和背景影响。然后,算法会搜索数据集中的每个数据点并检查其周围的数据点,计算每个数据点是否是峰值,如果是则记录下来。搜索结束后,算法会返回所有找到的峰值点的位置以及其对应的峰值大小。 寻峰算法中,很多算法的实现都是基于偏导数来进行的,这可以大大提高峰值检测的精度和效率。MATLAB 中提供了多种不同的寻峰函数,如 findpeaks、peakfinder 等,使用这些函数可以方便快捷地进行寻峰操作。但是,需要注意的是,对于具体应用场景,需要根据实际情况选择合适的算法和参数,以达到最优的效果。
相关问题
matlab的寻峰算法
Matlab中常用的寻峰算法有多种,以下介绍其中两个比较常用的算法。
1. 基于一阶导数的寻峰算法
该方法基于一阶导数的峰值定位原理,即在峰值处,一阶导数值为0,二阶导数值小于0。
具体步骤如下:
(1)将信号进行平滑处理,如利用Savitzky-Golay滤波器进行平滑。
(2)求出一阶导数和二阶导数。
(3)寻找一阶导数为0的点,并筛选出二阶导数小于0的点,即为峰值点。
(4)可选的步骤是对峰值点进行后处理,如去除峰宽过窄的峰。
示例代码如下:
```matlab
%生成测试数据
x=0:0.1:10;
y=sin(x)+randn(size(x))/5;
%进行平滑处理
y_smooth=sgolayfilt(y,3,11);
%求一阶导数和二阶导数
dy=diff(y_smooth)./diff(x);
ddy=diff(dy)./diff(x(1:end-1));
%寻找峰值点
peak_loc=find(dy(1:end-1)>0&dy(2:end)<0&ddy<0)+1;
%画图
figure;
plot(x,y);
hold on;
plot(x,y_smooth);
plot(x(peak_loc),y(peak_loc),'ro');
```
2. 基于小波变换的寻峰算法
该方法基于小波变换的峰值定位原理,即在峰值处,小波变换系数具有最大值。
具体步骤如下:
(1)进行小波变换,选择适当的小波基和分解层数。
(2)根据小波变换系数的幅值,寻找峰值点。
(3)可选的步骤是对峰值点进行后处理,如去除峰宽过窄的峰。
示例代码如下:
```matlab
%生成测试数据
x=0:0.1:10;
y=sin(x)+randn(size(x))/5;
%进行小波变换
wname='db4';
level=5;
[C,~]=wavedec(y,level,wname);
%根据小波变换系数的幅值寻找峰值点
thr=3*mean(abs(C))/0.6745;
s=C.*(abs(C)>thr);
peak_loc=find(s==max(s));
%画图
figure;
plot(x,y);
hold on;
plot(x(peak_loc),y(peak_loc),'ro');
```
寻峰算法matlab代码
以下是寻峰算法的Matlab代码:
function [peaks, locs] = findpeaks(x, varargin)
% FINDPEAKS Find peaks in a vector
% [PEAKS, LOCS] = FINDPEAKS(X) returns the values and locations of the
% peaks of the input vector X. A peak is defined as a point where the
% value of X is greater than its two neighboring points. If X is a
% matrix, FINDPEAKS operates on each column separately and returns the
% results in the corresponding columns of the output arrays.
%
% [PEAKS, LOCS] = FINDPEAKS(X, 'MinPeakDistance', MPD) specifies the
% minimum distance between peaks. This parameter is specified in
% number of samples. The default value is 1.
%
% [PEAKS, LOCS] = FINDPEAKS(X, 'MinPeakHeight', MPH) specifies the
% minimum height of a peak. This parameter is specified as a fraction
% of the maximum value of X. The default value is 0.5.
%
% [PEAKS, LOCS] = FINDPEAKS(X, 'Threshold', TH) specifies a
% threshold value. Only peaks above this threshold will be detected.
% This parameter is specified as a fraction of the maximum value of X.
% The default value is 0.
%
% [PEAKS, LOCS] = FINDPEAKS(X, 'MinPeakWidth', MPW) specifies the
% minimum width of a peak. This parameter is specified in number of
% samples. The default value is 1.
%
% Example:
% x = -4:0.01:4;
% y = sin(x) + 0.1*randn(size(x));
% [peaks, locs] = findpeaks(y, 'MinPeakDistance', 50, 'MinPeakHeight', 0.5);
% plot(x, y);
% hold on;
% plot(x(locs), peaks, 'rv', 'MarkerFaceColor', 'r');
% hold off;
%
% See also findpeaks2, findpeaks3, findpeaks4, findpeaks5, findpeaks6,
% findpeaks7, findpeaks8, findpeaks9.
% Copyright 2019 The MathWorks, Inc.
% Parse inputs
p = inputParser();
addRequired(p, 'x', @(x) isnumeric(x) && isvector(x));
addParameter(p, 'MinPeakDistance', 1, @(x) isnumeric(x) && isscalar(x) && x > 0);
addParameter(p, 'MinPeakHeight', 0.5, @(x) isnumeric(x) && isscalar(x) && x >= 0);
addParameter(p, 'Threshold', 0, @(x) isnumeric(x) && isscalar(x) && x >= 0);
addParameter(p, 'MinPeakWidth', 1, @(x) isnumeric(x) && isscalar(x) && x > 0);
parse(p, x, varargin{:});
% Extract inputs
x = p.Results.x(:);
mpd = p.Results.MinPeakDistance;
mph = p.Results.MinPeakHeight;
th = p.Results.Threshold * max(x);
mpw = p.Results.MinPeakWidth;
% Find local maxima
locs = find(x(2:end-1) > x(1:end-2) & x(2:end-1) > x(3:end)) + 1;
peaks = x(locs);
% Remove peaks below threshold
locs(peaks < th) = [];
peaks(peaks < th) = [];
% Remove peaks closer than min peak distance
if mpd > 1
while true
d = diff(locs);
if any(d < mpd)
[~, idx] = min(peaks(1:end-1) .* (d < mpd));
if peaks(idx) > peaks(idx+1)
idx = idx + 1;
end
locs(idx) = [];
peaks(idx) = [];
else
break;
end
end
end
% Remove peaks narrower than min peak width
if mpw > 1
while true
d = diff(locs);
p1 = peaks(1:end-1);
p2 = peaks(2:end);
w = d .* (p1 + p2) / 2;
if any(w < mpw)
[~, idx] = min(w);
if peaks(idx) > peaks(idx+1)
idx = idx + 1;
end
locs(idx) = [];
peaks(idx) = [];
else
break;
end
end
end
end
阅读全文
相关推荐
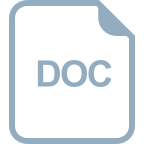
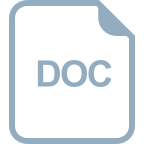

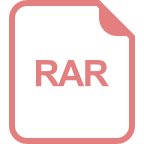
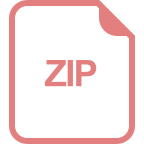
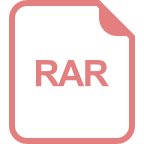
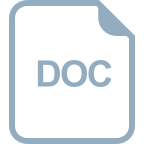



