cannot convert ‘node’ to ‘int’ in initialization
时间: 2024-05-06 16:17:20 浏览: 4
这个错误通常是因为你在代码中将一个非 int 类型的变量或对象赋值给了 int 类型的变量,导致编译器无法将其自动转换为 int 类型。
你可以检查一下代码中的变量类型是否正确,或者考虑使用类型转换函数将其转换为 int 类型。
例如,如果你想将一个字符串类型的变量 str 转换为 int 类型,可以使用 std::stoi 函数:
```
std::string str = "123";
int num = std::stoi(str);
```
注意:在使用 stoi 函数时,需要包含头文件 <string>。
相关问题
cannot convert 'Book' to 'Node' in initialization
This error occurs because you are trying to convert an object of type `Book` to an object of type `Node` during initialization. The compiler is indicating that this conversion is not possible.
To resolve this issue, you need to ensure that the types are compatible for the initialization. If `Book` is a subclass of `Node`, you can perform an upcasting to convert the `Book` object to a `Node` object.
Here is an example:
```cpp
class Node {
// ...
};
class Book : public Node {
// ...
};
Book book;
Node node = static_cast<Node>(book); // upcasting
```
In this example, the `Book` class is derived from the `Node` class. By using the `static_cast` operator, you can safely convert the `Book` object `book` to a `Node` object `node`.
Please note that this approach assumes that the `Book` class is indeed a subclass of the `Node` class. If they are unrelated or the inheritance relationship is not established correctly, you may need to reconsider your design or provide more context for further assistance.
[Error] cannot convert 'int*' to 'double' in initialization
这个错误通常是因为您将一个整数类型的指针赋值给了一个实数类型的变量。请确保您所赋值的类型与目标变量的类型相匹配。如果您需要将整数类型的指针转换为实数类型,请使用类型转换函数,如`static_cast`或`reinterpret_cast`。例如:
```
int* ptr = new int(10);
double num = static_cast<double>(*ptr);
```
这样就可以将指针`ptr`指向的整数类型值转换为双精度浮点数类型,并赋值给`num`。
相关推荐
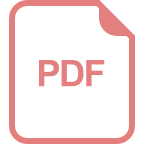
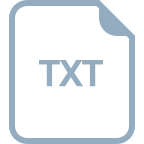












