请用python帮我写一个可以记录每天工作内容的脚本
时间: 2024-02-18 17:06:32 浏览: 21
好的,以下是一个简单的Python脚本,可以用于记录每天工作内容:
```python
import datetime
# 获取当天日期
today = datetime.date.today()
# 打开当天的工作日志文件,如果不存在则创建
with open(str(today) + '.txt', 'a') as f:
pass
# 输入当天工作内容,并写入日志文件
work_content = input('请输入今天的工作内容:')
with open(str(today) + '.txt', 'a') as f:
f.write(work_content + '\n')
```
运行这个脚本后,会要求你输入今天的工作内容,然后自动将其写入以当天日期命名的文本文件中。如果该文件不存在,则会自动创建。
你可以在每天结束后运行这个脚本,记录当天的工作内容。之后,你可以随时查阅这些日志文件,回顾自己的工作内容,或者进行其他操作。
相关问题
帮我写一个python脚本,能每天下午五点删除当前目录下超过50mb的文件,并用一个txt文件记录删除的文件名
我可以回答这个问题。以下是一个示例Python脚本,可以实现每天下午五点删除当前目录下超过50MB的文件,并将删除的文件名记录在一个txt文件中:
```python
import os
import datetime
# 获取当前时间
now = datetime.datetime.now()
# 判断是否为下午五点
if now.hour == 17:
# 获取当前目录
path = os.getcwd()
# 遍历当前目录下的文件
for file in os.listdir(path):
# 获取文件大小
size = os.path.getsize(os.path.join(path, file))
# 判断文件大小是否超过50MB
if size > 50 * 1024 * 1024:
# 删除文件
os.remove(os.path.join(path, file))
# 记录删除的文件名
with open('deleted_files.txt', 'a') as f:
f.write(file + '\n')
```
请注意,这只是一个示例脚本,您需要根据自己的实际情况进行修改和调整。同时,删除文件是一项危险的操作,请谨慎使用。
编写一个shell脚本,定时执行另一个python脚本
编写一个shell脚本,定时执行另一个python脚本可以通过使用crontab来实现。crontab是一个用于在Linux系统中定时执行任务的工具。下面是一个示例的shell脚本,用于定时执行另一个python脚本:
```shell
#!/bin/bash
# 设置定时任务
# 每天的8点执行python脚本
# 0 8 * * * 表示每天的8点
# /path/to/python 表示你的python解释器的路径
# /path/to/script.py 表示你要执行的python脚本的路径
# >> /path/to/log.txt 表示将输出重定向到一个日志文件中
echo "0 8 * * * /path/to/python /path/to/script.py >> /path/to/log.txt" | crontab -
```
请注意,你需要将`/path/to/python`替换为你的python解释器的路径,将`/path/to/script.py`替换为你要执行的python脚本的路径,将`/path/to/log.txt`替换为你想要将输出重定向到的日志文件的路径。
这样,每天的8点,crontab会自动执行指定的python脚本,并将输出记录到日志文件中。
相关推荐
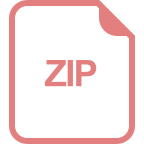
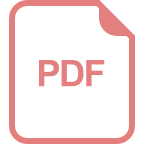
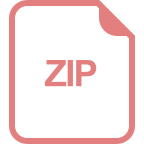












