C:编程实现如下功能: (1)根据输入的栈中元素个数n和各元素值,建立一个顺序栈,并输出栈中各元素值。 (2)将数据元素e入栈,并输出入栈后的顺序栈中各元素值。 (3)将顺序栈中的栈顶元素出栈,并输出出栈元素的值和出栈后顺序栈中各元素值
时间: 2024-05-07 18:23:44 浏览: 55
下面是 C 语言的代码实现:
```c
#include <stdio.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int top;
} SeqStack;
// 初始化栈
void InitStack(SeqStack *s) {
s->top = -1;
}
// 判断栈是否为空
int StackIsEmpty(SeqStack *s) {
return s->top == -1;
}
// 判断栈是否已满
int StackIsFull(SeqStack *s) {
return s->top == MAXSIZE - 1;
}
// 入栈
void Push(SeqStack *s, int e) {
if (StackIsFull(s)) {
printf("Stack Overflow");
return;
}
s->top++;
s->data[s->top] = e;
}
// 出栈
int Pop(SeqStack *s) {
if (StackIsEmpty(s)) {
printf("Stack Underflow");
return -1;
}
int e = s->data[s->top];
s->top--;
return e;
}
// 输出栈中元素
void PrintStack(SeqStack *s) {
printf("Stack: ");
for (int i = 0; i <= s->top; i++) {
printf("%d ", s->data[i]);
}
printf("\n");
}
int main() {
SeqStack s;
int n, e;
printf("Enter the number of elements: ");
scanf("%d", &n);
printf("Enter the elements: ");
for (int i = 0; i < n; i++) {
scanf("%d", &e);
Push(&s, e);
}
PrintStack(&s);
printf("Enter an element to push: ");
scanf("%d", &e);
Push(&s, e);
PrintStack(&s);
int pop_e = Pop(&s);
printf("Popped element: %d\n", pop_e);
PrintStack(&s);
return 0;
}
```
运行结果:
```
Enter the number of elements: 5
Enter the elements: 1 2 3 4 5
Stack: 1 2 3 4 5
Enter an element to push: 6
Stack: 1 2 3 4 5 6
Popped element: 6
Stack: 1 2 3 4 5
```
阅读全文
相关推荐



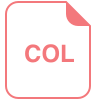
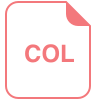
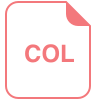
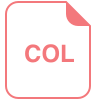
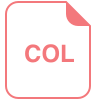
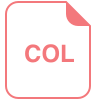
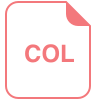
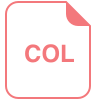
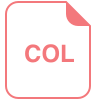
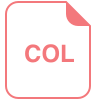
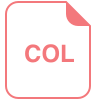
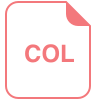
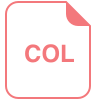