MAX31856的STM32代码
时间: 2024-03-20 13:16:56 浏览: 139
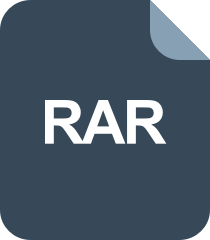
MAX31856的STM32代码,完整,好用,注释清晰,本人原创。还有中文资料和原理图。

由于MAX31856是一款数字温度传感器,因此它只需使用SPI接口与STM32通信。以下是一份简单的STM32代码:
```c
#include "stm32f10x.h"
#include "spi.h"
#include "max31856.h"
#define MAX31856_CS_PIN GPIO_Pin_4
#define MAX31856_CS_PORT GPIOA
void MAX31856_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
SPI_InitTypeDef SPI_InitStructure;
// Enable GPIO clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// Enable SPI clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
// Configure CS pin
GPIO_InitStructure.GPIO_Pin = MAX31856_CS_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(MAX31856_CS_PORT, &GPIO_InitStructure);
// Configure SPI pins
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure SPI
SPI_InitStructure.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStructure.SPI_CRCPolynomial = 7;
SPI_Init(SPI1, &SPI_InitStructure);
// Enable SPI
SPI_Cmd(SPI1, ENABLE);
}
uint8_t MAX31856_ReadRegister(uint8_t reg)
{
uint8_t data;
// Set CS low
GPIO_ResetBits(MAX31856_CS_PORT, MAX31856_CS_PIN);
// Send read command
SPI_I2S_SendData(SPI1, (reg << 2) | 0x01);
// Wait for transfer to complete
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
// Receive data
data = SPI_I2S_ReceiveData(SPI1);
// Set CS high
GPIO_SetBits(MAX31856_CS_PORT, MAX31856_CS_PIN);
return data;
}
void MAX31856_WriteRegister(uint8_t reg, uint8_t value)
{
// Set CS low
GPIO_ResetBits(MAX31856_CS_PORT, MAX31856_CS_PIN);
// Send write command
SPI_I2S_SendData(SPI1, (reg << 2) & 0xFC);
// Wait for transfer to complete
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
// Send data
SPI_I2S_SendData(SPI1, value);
// Wait for transfer to complete
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
// Set CS high
GPIO_SetBits(MAX31856_CS_PORT, MAX31856_CS_PIN);
}
float MAX31856_ReadTemperature(void)
{
uint8_t reg = MAX31856_REG_RTD_MSB;
uint8_t data[4];
int32_t rtd, ref, rtd_scaled, ref_scaled;
float resistance, temperature;
// Read RTD and reference data
for (int i = 0; i < 4; i++) {
data[i] = MAX31856_ReadRegister(reg++);
}
// Combine RTD and reference data
rtd = ((int32_t)data[0] << 8) | data[1];
ref = ((int32_t)data[2] << 8) | data[3];
// Scale RTD and reference values
rtd_scaled = rtd >> 1;
ref_scaled = ref >> 1;
// Calculate resistance
resistance = ((float)ref_scaled / (float)32768.0) * 430.0;
// Calculate temperature
temperature = (resistance - 100.0) / 0.385;
return temperature;
}
```
在上面的代码中,我们首先配置了GPIO和SPI,并实现了一个用于读取和写入MAX31856寄存器的函数。然后,我们实现了一个函数来读取传感器的温度值。这个函数首先读取MAX31856的RTD和参考数据,然后使用这些数据来计算温度。注意,这个函数返回的是温度值,而不是原始的RTD或参考数据。
阅读全文
相关推荐
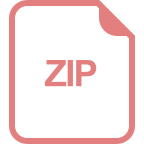




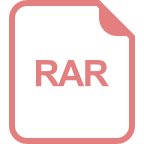
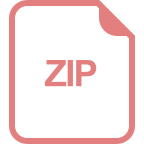
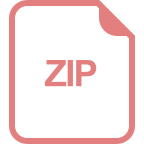
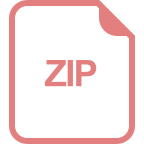
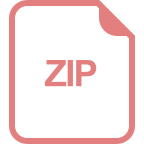
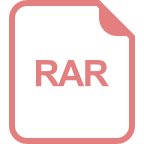
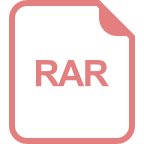
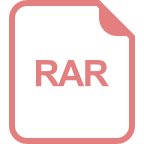
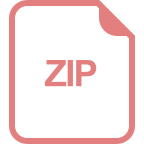

