使用python编写程序实现aes加密解密
时间: 2023-07-20 17:16:50 浏览: 170
好的,以下是一个使用Python的PyCryptodome库实现AES加密和解密的示例代码:
```python
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
import base64
def encryptAES(plainText, key):
# 将密钥转换为bytes类型
key = key.encode('utf-8')
# 初始化加密器,选择ECB加密模式
cipher = AES.new(key, AES.MODE_ECB)
# 加密
cipherText = cipher.encrypt(pad(plainText.encode('utf-8'), AES.block_size))
# 返回Base64编码后的密文
return base64.b64encode(cipherText).decode('utf-8')
def decryptAES(cipherText, key):
# 将密钥转换为bytes类型
key = key.encode('utf-8')
# 初始化解密器,选择ECB加密模式
cipher = AES.new(key, AES.MODE_ECB)
# 解密
plainText = unpad(cipher.decrypt(base64.b64decode(cipherText.encode('utf-8'))), AES.block_size)
# 返回明文
return plainText.decode('utf-8')
# 测试
plainText = "Hello, world!"
key = "1234567890123456"
cipherText = encryptAES(plainText, key)
print("Cipher text:", cipherText)
decryptedText = decryptAES(cipherText, key)
print("Decrypted text:", decryptedText)
```
这个示例代码使用了PyCryptodome库提供的AES加密和解密函数,使用ECB加密模式进行加密和解密,返回的是Base64编码后的密文。你可以根据自己的需求修改加密模式和返回结果的格式。
阅读全文
相关推荐
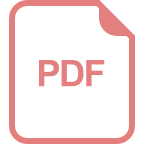
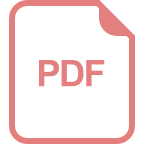
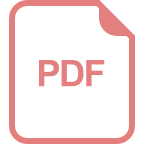
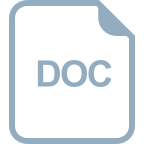



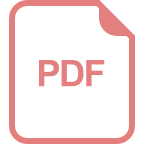
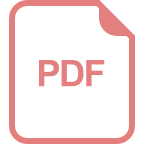
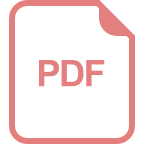
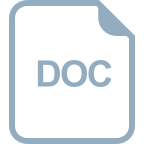
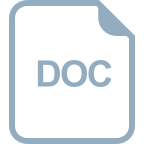
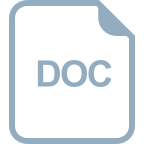
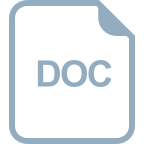
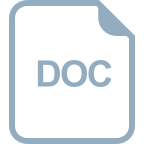
