Python实现AES加密解密算法程序
时间: 2023-11-05 19:14:05 浏览: 136
好的,下面是Python实现AES加密解密算法程序的示例代码:
```python
import base64
from Crypto.Cipher import AES
# 定义加密函数
def aes_encrypt(key, data):
# 将密钥和数据分别进行base64编码
key = base64.b64encode(key.encode('utf-8')).decode('utf-8')
data = base64.b64encode(data.encode('utf-8')).decode('utf-8')
# 使用CBC模式加密
cipher = AES.new(key.encode('utf-8'), AES.MODE_CBC, b'0000000000000000')
# 加密数据
encrypted_data = cipher.encrypt(data.encode('utf-8'))
# 将加密后的数据进行base64编码
encrypted_data = base64.b64encode(encrypted_data).decode('utf-8')
return encrypted_data
# 定义解密函数
def aes_decrypt(key, encrypted_data):
# 将密钥进行base64编码
key = base64.b64encode(key.encode('utf-8')).decode('utf-8')
# 将加密后的数据进行base64解码
encrypted_data = base64.b64decode(encrypted_data)
# 使用CBC模式解密
cipher = AES.new(key.encode('utf-8'), AES.MODE_CBC, b'0000000000000000')
# 解密数据
decrypted_data = cipher.decrypt(encrypted_data).decode('utf-8')
# 将解密后的数据进行base64解码
decrypted_data = base64.b64decode(decrypted_data).decode('utf-8')
return decrypted_data
# 测试加密解密函数
if __name__ == '__main__':
key = '1234567890abcdef' # 密钥长度必须为16、24或32个字符
data = '这是一条测试数据'
encrypted_data = aes_encrypt(key, data)
print('加密后的数据:', encrypted_data)
decrypted_data = aes_decrypt(key, encrypted_data)
print('解密后的数据:', decrypted_data)
```
需要注意的是,上述示例代码中使用的是AES加密算法中的CBC模式,密钥长度必须为16、24或32个字符。如果需要使用其他模式或密钥长度,需要根据具体情况进行修改。
阅读全文
相关推荐
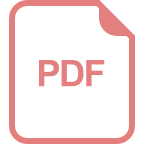
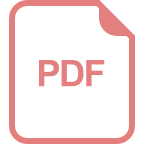


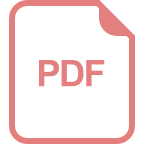
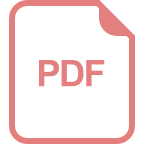
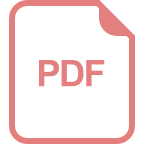
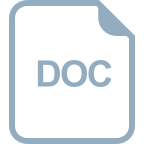
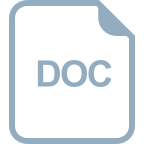
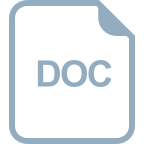
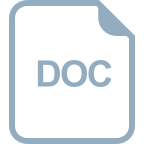




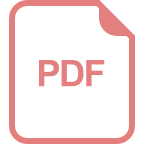