用C++实习将三维直角坐标下的位置速度转换为柱坐标下的位置速度
时间: 2024-03-27 07:36:55 浏览: 15
要将三维直角坐标系下的位置速度转换为柱坐标系下的位置速度,可以按照以下步骤进行:
1. 首先,计算出点在三维直角坐标系下的极径r、极角θ和高度z,公式如下:
r = sqrt(x^2 + y^2)
θ = atan2(y, x)
z = z
其中,x、y、z分别为点在三维直角坐标系下的坐标。
2. 接着,计算出点在柱坐标系下的速度向量的大小和方向。速度向量的大小可以通过三维直角坐标系下的速度向量的x、y、z分量计算得到:
v = sqrt(vx^2 + vy^2 + vz^2)
其中,vx、vy、vz分别为点在三维直角坐标系下的速度向量分量。
速度向量的方向可以通过极角θ和高度z计算得到:
vθ = (vx * cos(θ) + vy * sin(θ)) / r
vz = vz
vφ = (-vx * sin(θ) + vy * cos(θ)) / r
其中,vθ、vφ、vz分别为点在柱坐标系下的速度向量在极角θ、方位角φ和高度z方向上的分量。
3. 最后,将计算得到的柱坐标系下的位置和速度向量分量存储起来即可。
下面是一个用C++实现的将三维直角坐标系下的位置速度转换为柱坐标系下的位置速度的示例代码:
```cpp
#include <cmath>
// 定义一个结构体表示点在三维直角坐标系下的位置和速度
struct CartesianCoordinate {
double x, y, z;
double vx, vy, vz;
};
// 定义一个结构体表示点在柱坐标系下的位置和速度
struct CylindricalCoordinate {
double r, theta, z;
double vr, vtheta, vz;
};
// 将点在三维直角坐标系下的位置速度转换为柱坐标系下的位置速度
CylindricalCoordinate cartesianToCylindrical(const CartesianCoordinate& cartesian) {
CylindricalCoordinate cylindrical;
// 计算极径r、极角theta和高度z
cylindrical.r = sqrt(cartesian.x * cartesian.x + cartesian.y * cartesian.y);
cylindrical.theta = atan2(cartesian.y, cartesian.x);
cylindrical.z = cartesian.z;
// 计算速度向量的大小和方向
double v = sqrt(cartesian.vx * cartesian.vx + cartesian.vy * cartesian.vy + cartesian.vz * cartesian.vz);
cylindrical.vr = (cartesian.x * cartesian.vx + cartesian.y * cartesian.vy) / cylindrical.r;
cylindrical.vtheta = (cartesian.vx * cos(cylindrical.theta) + cartesian.vy * sin(cylindrical.theta)) / cylindrical.r;
cylindrical.vz = cartesian.vz;
return cylindrical;
}
```
相关推荐
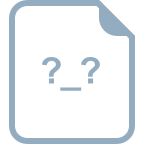














