以函数的形式实现,并用建立新的线程方式监测/sys/class/gpio/gpio2是否有中断发生C语言,中断触发条件可以选rising,fallin,both
时间: 2024-01-09 15:03:51 浏览: 88
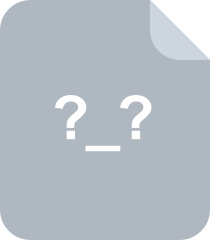
中断的实现(可以计算中断次数并在屏幕上显示)

以下是一个以函数形式实现并用新线程监测GPIO中断的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/select.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <sys/time.h>
#define GPIO_PIN 2
#define GPIO_DIR "/sys/class/gpio/gpio2/"
#define GPIO_VALUE GPIO_DIR "value"
#define GPIO_EDGE GPIO_DIR "edge"
void *gpio_monitor(void *arg) {
fd_set fds;
struct timeval tv;
int fd, ret, val;
char buf[8];
fd = open(GPIO_VALUE, O_RDONLY);
if (fd < 0) {
perror("open");
return NULL;
}
while (1) {
FD_ZERO(&fds);
FD_SET(fd, &fds);
tv.tv_sec = 1;
tv.tv_usec = 0;
ret = select(fd + 1, &fds, NULL, NULL, &tv);
if (ret < 0) {
perror("select");
break;
} else if (ret == 0) {
continue;
} else {
lseek(fd, 0, SEEK_SET);
ret = read(fd, buf, sizeof(buf));
if (ret < 0) {
perror("read");
break;
}
val = atoi(buf);
printf("GPIO value: %d\n", val);
}
}
close(fd);
return NULL;
}
int gpio_init() {
int fd;
char buf[8];
fd = open(GPIO_DIR "direction", O_WRONLY);
if (fd < 0) {
perror("open");
return -1;
}
sprintf(buf, "in");
write(fd, buf, sizeof(buf));
close(fd);
fd = open(GPIO_EDGE, O_WRONLY);
if (fd < 0) {
perror("open");
return -1;
}
sprintf(buf, "both");
write(fd, buf, sizeof(buf));
close(fd);
return 0;
}
int gpio_start_monitor() {
pthread_t tid;
int ret;
ret = pthread_create(&tid, NULL, gpio_monitor, NULL);
if (ret != 0) {
perror("pthread_create");
return -1;
}
return 0;
}
int main() {
int ret;
ret = gpio_init();
if (ret != 0) {
printf("Failed to initialize GPIO\n");
return -1;
}
ret = gpio_start_monitor();
if (ret != 0) {
printf("Failed to start GPIO monitor\n");
return -1;
}
printf("GPIO monitor started\n");
while (1) {
sleep(1);
}
return 0;
}
```
该程序将GPIO2作为输入引脚,设置其中断触发条件为上升沿、下降沿和边沿,然后在一个新线程中监测GPIO的值。当GPIO的值发生变化时,该程序会输出变化后的值。注意,该程序仅在Linux系统下运行。
阅读全文
相关推荐
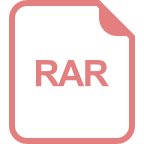
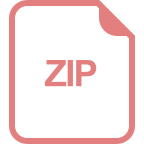

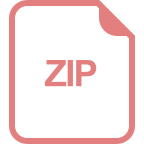
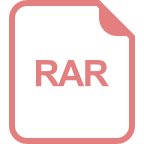
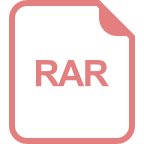
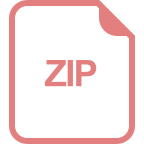
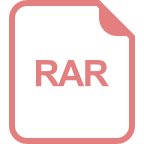
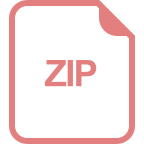
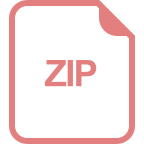
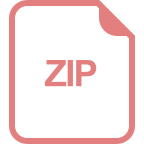
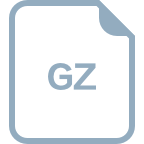
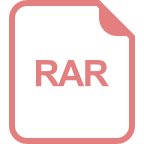
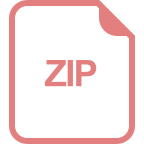
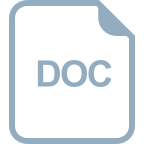
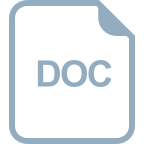