fastapi判断用户是否第一次访问
时间: 2023-08-03 07:04:01 浏览: 206
要判断用户是否第一次访问,可以为每个用户设置一个唯一的标识符,例如使用浏览器中的cookie或者在服务器端使用session。使用FastAPI,可以通过以下方式获取和设置cookie和session:
获取cookie:
```python
from fastapi import FastAPI, Request
app = FastAPI()
@app.get("/")
async def read_root(request: Request):
cookie_value = request.cookies.get("user_id")
if cookie_value:
return {"message": "Welcome back!"}
else:
return {"message": "Welcome for the first time!"}
```
设置cookie:
```python
from fastapi import FastAPI, Response
app = FastAPI()
@app.get("/")
async def read_root(response: Response):
response.set_cookie(key="user_id", value="unique-user-id")
return {"message": "Cookie set!"}
```
设置session:
```python
from fastapi import FastAPI, Request, Response, Depends
from fastapi.security import HTTPBasic, HTTPBasicCredentials
from fastapi.responses import RedirectResponse
from fastapi.templating import Jinja2Templates
from typing import Optional
app = FastAPI()
security = HTTPBasic()
templates = Jinja2Templates(directory="templates")
fake_users_db = {
"johndoe": {
"username": "johndoe",
"full_name": "John Doe",
"email": "johndoe@example.com",
"hashed_password": "fakehashedsecret",
"disabled": False,
},
"alice": {
"username": "alice",
"full_name": "Alice Wonderson",
"email": "alice@example.com",
"hashed_password": "fakehashedsecret2",
"disabled": True,
},
}
def get_current_user(session_token: Optional[str] = None):
if not session_token:
return None
username = session_token.split(":")[0]
user = fake_users_db.get(username)
if user:
return user
return None
@app.get("/")
async def read_root(request: Request, current_user: Optional[dict] = Depends(get_current_user)):
if current_user:
return {"message": "Welcome back, " + current_user["full_name"] + "!"}
else:
return {"message": "Welcome for the first time!"}
@app.post("/login")
async def login(response: Response, credentials: HTTPBasicCredentials = Depends(security)):
correct_username = credentials.username in fake_users_db
correct_password = False
if correct_username:
user = fake_users_db[credentials.username]
correct_password = credentials.password == user["hashed_password"]
if not (correct_username and correct_password):
return {"error": "Incorrect username or password", "response": response}
session_token = credentials.username + ":" + credentials.password
response.set_cookie(key="session_token", value=session_token)
return RedirectResponse(url="/")
@app.post("/logout")
async def logout(response: Response):
response.delete_cookie(key="session_token")
return RedirectResponse(url="/")
```
在这个示例中,如果用户登录成功,会设置一个名为session_token的cookie,其中保存了用户名和密码的组合。然后在每次请求时,都会检查该cookie是否存在,并且使用该cookie中的信息来识别当前用户。
阅读全文
相关推荐
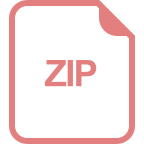
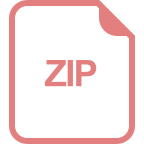



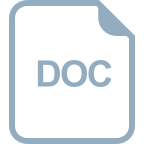
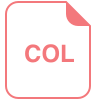
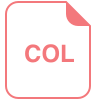
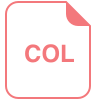
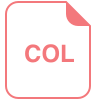
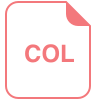
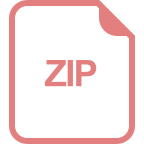
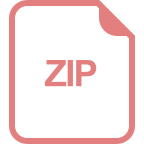
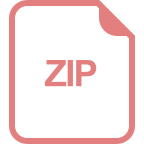
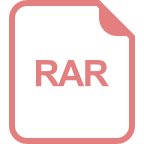
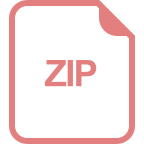