c语言将文件导入代码中,结构体数组
时间: 2024-02-28 07:54:45 浏览: 81
你可以使用C语言中的文件操作函数将文件导入代码中。一种常见的方法是使用fscanf函数逐行读取文件内容,并将其存储到结构体数组中。
下面是一个示例代码,其中假设文件中每行包含三个字段:name、age和gender,以空格分隔。
```c
#include <stdio.h>
struct Person {
char name[20];
int age;
char gender[10];
};
int main() {
struct Person people[100];
int count = 0;
FILE *file = fopen("people.txt", "r"); // 打开文件
if (file == NULL) {
printf("Failed to open file!");
return 1;
}
while (!feof(file)) { // 逐行读取文件内容
fscanf(file, "%s %d %s", people[count].name, &people[count].age, people[count].gender);
count++;
}
fclose(file); // 关闭文件
// 输出读取到的内容
for (int i = 0; i < count; i++) {
printf("Name: %s, Age: %d, Gender: %s\n", people[i].name, people[i].age, people[i].gender);
}
return 0;
}
```
在上面的示例代码中,我们首先定义了一个名为Person的结构体,包含三个字段:name、age和gender。然后,我们声明了一个包含100个Person结构体的数组,用于存储从文件中读取的内容。
接着,我们使用fopen函数打开文件,并使用fscanf函数逐行读取文件内容。每次读取一行后,我们将其存储到结构体数组中,并增加计数器count的值,以便后续输出使用。
最后,我们使用fclose函数关闭文件,并输出读取到的内容。
需要注意的是,以上示例代码只是一个简单的例子,实际使用中还需要考虑文件不存在、读取格式错误等异常情况。
阅读全文
相关推荐








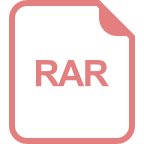








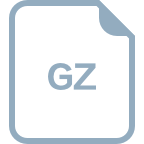
