基于ssm用jsp和ajax写一个文件上传案例
时间: 2024-02-12 22:08:27 浏览: 24
好的,以下是一个基于 SSM(Spring+SpringMVC+MyBatis)框架,使用 JSP 和 AJAX 实现文件上传的案例。
1. 在 SpringMVC 配置文件中添加文件上传解析器:
```xml
<!-- 配置文件上传解析器 -->
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="maxUploadSize" value="10485760"/> <!-- 限制上传文件的大小为10MB -->
</bean>
```
2. 在 JSP 页面中添加表单和 AJAX 代码:
```html
<form id="uploadForm" enctype="multipart/form-data">
<input type="file" name="file"/>
<input type="text" name="name"/>
<button id="uploadBtn" type="button">上传</button>
</form>
<script>
$(function() {
$('#uploadBtn').click(function() {
var formData = new FormData($('#uploadForm')[0]);
$.ajax({
url: 'upload',
type: 'POST',
data: formData,
processData: false,
contentType: false,
xhr: function() { // 监听上传进度
var xhr = $.ajaxSettings.xhr();
if (xhr.upload) {
xhr.upload.addEventListener('progress', function(event) {
if (event.lengthComputable) {
var percent = Math.round((event.loaded / event.total) * 100);
console.log('上传进度:' + percent + '%');
}
}, false);
}
return xhr;
},
success: function(result) {
console.log('上传成功');
},
error: function() {
console.log('上传失败');
}
});
});
});
</script>
```
3. 在 SpringMVC 控制器中添加文件上传处理方法:
```java
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ResponseBody
public String upload(@RequestParam("file") MultipartFile file, @RequestParam("name") String name) {
try {
if (!file.isEmpty()) {
String fileName = file.getOriginalFilename();
String filePath = "/data/" + fileName; // 上传文件保存路径
File dest = new File(filePath);
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
file.transferTo(dest);
// TODO: 处理上传文件和其他参数
return "success";
}
} catch (Exception e) {
e.printStackTrace();
}
return "error";
}
```
其中,`@RequestParam("file")` 注解表示接收名为 `file` 的文件参数,`@RequestParam("name")` 注解表示接收名为 `name` 的其他表单参数。
以上就是一个简单的基于 SSM、JSP 和 AJAX 的文件上传案例。注意,为了方便起见,这里使用了 jQuery 库来简化 AJAX 代码的编写。
相关推荐
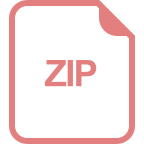
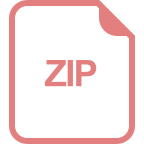
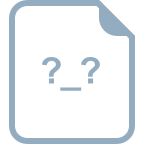
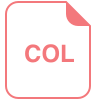
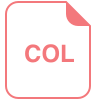
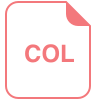
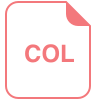
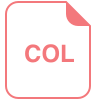









