根据极坐标下的png毫米波雷达数据,和标注出目标的二维边界框信息,根据毫米波雷达的强度,来计算目标的高度,给出具体代码
时间: 2023-11-08 15:05:34 浏览: 215
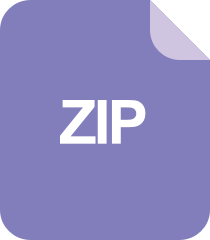
毫米波雷达的系统设计细节(2)-关于目标RCS的问题博文相对应的代码和数据
计算目标高度的代码需要涉及到雷达数据的处理和目标边界框的提取。以下是一种可能的实现方式,需要使用Python和NumPy库:
首先,读取极坐标下的png毫米波雷达数据,将其转换为二维数组。可以使用Python的Pillow库来读取PNG文件,然后使用NumPy的reshape函数将像素数据转换为二维数组。
```python
from PIL import Image
import numpy as np
# 读取PNG文件并转换为NumPy数组
img = Image.open("radar.png")
data = np.array(img.getdata()).reshape(img.size[1], img.size[0])
```
接下来,根据雷达数据的强度,找出目标边界框的位置。可以使用NumPy的argwhere函数来找出强度大于某个阈值的像素的位置,并使用NumPy的min和max函数找出最小和最大的行和列值。
```python
# 找出强度大于阈值的像素的位置
threshold = 200
points = np.argwhere(data > threshold)
# 找出最小和最大的行和列值
min_row, min_col = np.min(points, axis=0)
max_row, max_col = np.max(points, axis=0)
```
现在已经得到了目标边界框的位置,可以根据目标边界框的大小和雷达的参数来计算目标的高度。假设雷达的角度范围为[-45, 45]度,距离范围为[0, 100]米,目标边界框的宽度为200个像素,高度为50个像素,那么可以按照以下方式计算目标的高度:
```python
# 雷达参数
angle_range = np.deg2rad(90) # 角度范围
distance_range = 100 # 距离范围
# 目标边界框大小
width = 200
height = 50
# 计算目标的高度
center_col = (min_col + max_col) / 2
theta = angle_range * (center_col / data.shape[1] - 0.5)
distance = distance_range * (max_row / data.shape[0])
height = height / data.shape[0] * distance / np.sin(theta)
```
这样就可以得到目标的高度。完整的代码如下:
```python
from PIL import Image
import numpy as np
# 读取PNG文件并转换为NumPy数组
img = Image.open("radar.png")
data = np.array(img.getdata()).reshape(img.size[1], img.size[0])
# 找出强度大于阈值的像素的位置
threshold = 200
points = np.argwhere(data > threshold)
# 找出最小和最大的行和列值
min_row, min_col = np.min(points, axis=0)
max_row, max_col = np.max(points, axis=0)
# 雷达参数
angle_range = np.deg2rad(90) # 角度范围
distance_range = 100 # 距离范围
# 目标边界框大小
width = 200
height = 50
# 计算目标的高度
center_col = (min_col + max_col) / 2
theta = angle_range * (center_col / data.shape[1] - 0.5)
distance = distance_range * (max_row / data.shape[0])
height = height / data.shape[0] * distance / np.sin(theta)
print("目标高度为:", height)
```
阅读全文
相关推荐
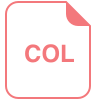
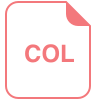
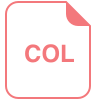
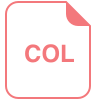
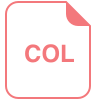
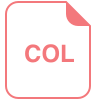
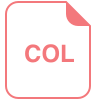
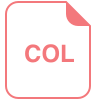
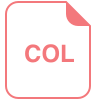
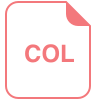
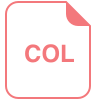
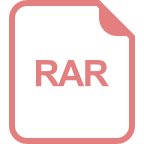
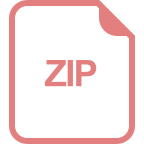