定义一个表示学生的类student,包括的成员变量有学号、姓名、性别、年龄,包括的成员方法有获得学号、姓名、性别、年龄和修改年龄,书写Java程序创建student类的对象及测试其方法的功能
时间: 2024-09-25 07:02:40 浏览: 26
定义一个`Student`类可以按照以下步骤进行:
```java
public class Student {
// 成员变量(属性)
private String id; // 学号
private String name; // 姓名
private String gender; // 性别
private int age; // 年龄
// 构造器(默认构造器)
public Student() {
this.id = "";
this.name = "";
this.gender = "";
this.age = 0;
}
// 获取成员变量的方法
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public int getAge() {
return age;
}
// 修改年龄的方法(设置方法)
public void setAge(int newAge) {
if (newAge >= 0) { // 验证年龄是否合法
age = newAge;
} else {
System.out.println("年龄不能为负数或零");
}
}
}
```
接下来,我们可以创建`Student`类的对象并测试它的方法:
```java
public class Main {
public static void main(String[] args) {
// 创建一个Student对象
Student student = new Student();
// 测试获取方法
System.out.println("初始学生信息: ");
System.out.println("学号: " + student.getId());
System.out.println("姓名: " + student.getName());
System.out.println("性别: " + student.getGender());
System.out.println("年龄: " + student.getAge());
// 测试修改年龄方法
student.setAge(20); // 设置年龄为20岁
System.out.println("\n修改后的学生信息: ");
System.out.println("年龄: " + student.getAge()); // 输出修改后的年龄
// 不合法年龄测试
student.setAge(-5);
}
}
```
在这个例子中,我们首先创建了一个`Student`对象,并使用默认构造器初始化了所有属性。然后,我们分别调用获取和设置方法来查看和改变学生的年龄。
相关推荐
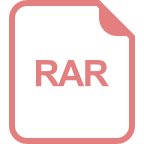
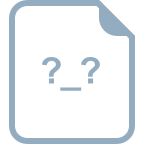
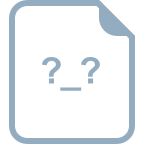














