return new String(Base64.getEncoder().encode(encryptedBytes));这段代码是什么意思?
时间: 2024-06-01 17:10:11 浏览: 84
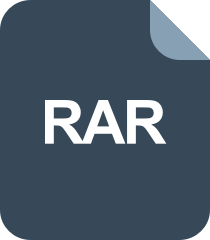
sun.misc.BASE64Encoder源码及jar包
这段代码将加密后的字节数组使用Base64编码成字符串。其中,Base64是一种编码方式,可以将二进制数据转换成可打印的ASCII字符,方便在网络上传输或存储。getEncoder()方法返回一个Base64编码器,encode()方法将字节数组进行编码,最后通过new String()方法将编码后的字节数组转换成字符串。
阅读全文
相关推荐
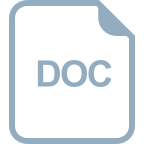
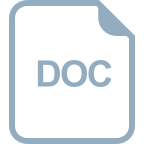
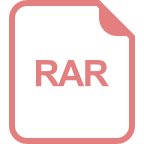
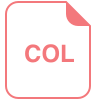
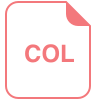




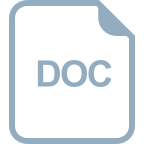
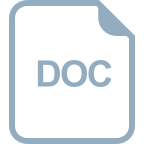
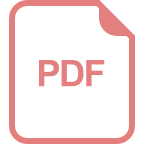
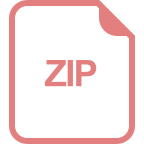
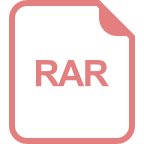
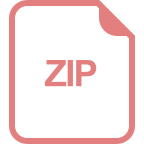
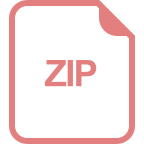
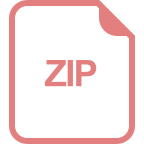
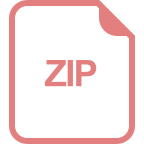