19. 加密保护技术应用案例研究
发布时间: 2024-01-27 10:47:02 阅读量: 17 订阅数: 16 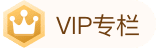
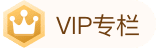
# 1. 加密保护技术概述
## 1.1 加密保护技术的定义和发展历程
加密保护技术是指通过对数据进行加密,以确保数据在传输或存储过程中不被未授权的个人或实体所访问、窃取或篡改的一种安全保护技术。随着信息技术的发展,加密保护技术得到了广泛应用,并逐渐形成了完整的理论体系和技术体系。
加密保护技术最初起源于军事领域,随着计算机和互联网技术的发展,逐渐渗透到了商业和个人领域,成为信息安全的重要组成部分。发展至今,加密保护技术已经非常成熟,涉及的领域也日益广泛,包括网络通信、金融交易、医疗健康、云计算等各个行业。
## 1.2 加密保护技术的基本原理
加密保护技术的基本原理包括对称加密和非对称加密两种方式。对称加密使用相同的密钥进行加密和解密,加密速度快,适合大数据量的加密,但密钥的安全传输是一个难题;非对称加密使用公钥和私钥进行加密和解密,安全性更高,但加密解密速度较慢,适合对安全性要求较高的场合。
## 1.3 加密保护技术在信息安全中的作用
加密保护技术在信息安全中起着至关重要的作用,它可以有效防止信息被窃取、篡改和伪造。在网络通信中,通过加密技术可以保障数据的机密性和完整性;在数据存储和传输过程中,加密技术可以保护数据的安全性;在云计算和大数据领域,加密技术可以有效保护大规模数据的安全。
以上是加密保护技术概述的内容,接下来我们将深入探讨加密保护技术的分类与应用。
# 2. 加密保护技术分类与应用
### 2.1 对称加密算法的案例研究
在信息安全领域,对称加密算法是一种常用的加密技术。它使用同一个密钥来进行加密和解密操作,速度较快,适用于对大量数据进行加密保护的场景。
#### 2.1.1 DES算法实现
DES(Data Encryption Standard)是一种对称加密算法,采用56位密钥进行加密和解密。下面是一个使用Python实现DES加密算法的示例代码:
```python
from Crypto.Cipher import DES
from Crypto.Util.Padding import pad, unpad
def encrypt_des(key, plaintext):
cipher = DES.new(key, DES.MODE_ECB)
padded_plaintext = pad(plaintext.encode(), DES.block_size)
ciphertext = cipher.encrypt(padded_plaintext)
return ciphertext.hex()
def decrypt_des(key, ciphertext):
cipher = DES.new(key, DES.MODE_ECB)
decrypted_data = cipher.decrypt(bytes.fromhex(ciphertext))
plaintext = unpad(decrypted_data, DES.block_size).decode()
return plaintext
# 示例演示
key = b'01234567'
plaintext = 'Hello, World!'
ciphertext = encrypt_des(key, plaintext)
decrypted_text = decrypt_des(key, ciphertext)
print('Plaintext:', plaintext)
print('Ciphertext:', ciphertext)
print('Decrypted Text:', decrypted_text)
```
代码解释:
- `encrypt_des`函数使用DES算法进行加密,接受一个密钥和明文参数,返回加密后的密文。
- `decrypt_des`函数使用DES算法进行解密,接受一个密钥和密文参数,返回解密后的明文。
- 示例演示使用了一个8字节的密钥和一段明文进行加密和解密操作。
#### 2.1.2 AES算法实现
AES(Advanced Encryption Standard)是一种高级的对称加密算法,使用多种密钥长度(128位、192位和256位)进行加密和解密。下面是一个使用Java实现AES加密算法的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class AESExample {
public static String encryptAES(String key, String plaintext) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedData = cipher.doFinal(plaintext.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedData);
}
public static String decryptAES(String key, String ciphertext) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedData = cipher.doFinal(Base64.getDecoder().decode(ciphertext));
return new String(decryptedData, StandardCharsets.UTF_8);
}
// 示例演示
public static void main(String[] args) throws Exception {
String key = "0123456789abcdef";
String plaintext = "Hello, World!";
String ciphertext = encryptAES(key, plaintext);
String decryptedText = decryptAES(key, ciphertext);
System.out.println("Plaintext: " + plaintext);
System.out.println("Ciphertext: " + ciphertext);
System.out.println("Decrypted Text: " + decryptedText);
}
}
```
代码解释:
- `encryptAES`方法使用AES算法进行加密,接受一个密钥和明文参数,返回加密后的Base64编码密文。
- `decryptAES`方法使用AES算法进行解密,接受一个密钥和Base64编码的密文参数,返回解密后的明文。
- 示例演示使用了一个16字节的密钥和一段明文进行加密和解密操作。
### 2.2 非对称加密算法的案例研究
非对称加密算法使用一对密钥(公钥和私钥)进行加密和解密操作,安全性更高,适用于密钥交换和数字签名等场景。
#### 2.2.1 RSA算法实现
RSA算法是一种常用的非对称加密算法,被广泛应用于数据加密和数字签名等领域。下面是一个使用Go语言实现RSA加密算法的示例代码:
```go
package main
import (
"crypto/rand"
"crypto/rsa"
"crypto/x509"
"encoding/base64"
"encoding/pem"
"fmt"
)
func rsaEncrypt(plainText []byte, publicKey *rsa.PublicKey) (string, error) {
ciphertext, err := rsa.EncryptPKCS1v15(rand.Reader, publicKey, plainText)
if err != nil {
return "", err
}
return base64.StdEncoding.EncodeToString(ciphertext), nil
}
func rsaDecrypt(ciphertext string, privateKey *rsa.PrivateKey) (string, error) {
encryptedData, err := base64.StdEncoding.DecodeString(ciphertext)
if err != nil {
return "", err
}
plaintext, err := rsa.DecryptPKCS1v15(rand.Reader, privateKey, encryptedData)
if err != nil {
return "", err
}
return string(plaintext), nil
}
func main() {
plainText := []byte("Hello, World!")
// 生成RSA密钥对
privateKey, _ := rsa.GenerateKey(rand.Reader, 2048)
publicKey := &privateKey.PublicKey
// RSA加密
ciphertext, _ := rsaEncrypt(plainText, publicKey)
// RSA解密
decryptedText, _ := rsaDecrypt(ciphertext, privateKey)
fmt.Println("Plaintext:", string(plainText))
fmt.Println("Ciphertext:", ciphertext)
fmt.Println("Decrypted Text:", decryptedText)
}
```
代码解释:
- `rsaEncrypt`函数使用公钥对明文进行加密,返回Base64编码的密文字符串。
- `rsaDecrypt`函数使用私钥对密文进行解密,返回解密后的明文字符串。
- 示例演示了RSA算法的使用,包括密钥生成、加密和解密操作。
### 2.3 哈希算法的案例研究
哈希算法是一种将任意长度的数据映射为固定长度的摘要信息的算法,常用于数据完整性校验和防篡改等场景。
#### 2.3.1 SHA-256算法实现
SHA-256(Secure Hash Algorithm 256-bit)是一种常用的哈希算法,其生成的摘要信息长度为256位。下面是一个使用JavaScript实现SHA-256哈希算法的示例代码:
```javascript
const crypto = require('crypto');
function sha256Hash(data) {
const hash = crypto.createHash('sha256');
hash.update(data);
return hash.digest('hex');
}
// 示例演示
const data = 'Hello, World!';
const hash = sha256Hash(data);
console.log('Data:', data);
console.log('Hash:', hash);
```
代码解释:
- `sha256Hash`函数使用Node.js内置的`crypto`模块创建SHA-256哈希对象,更新数据,并返回十六进制表示的摘要信息。
- 示例演示使用了一段数据进行SHA-256哈希计算。
### 2.4 加密保护技术在网络安全中的应用案例
加密保护技术在网络安全中起着重要的作用,确保网络通信的机密性和完整性。下面是一个使用Python实现加密传输的网络通信案例:
```python
import socket
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt_data(key, data):
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.enc
```
0
0
相关推荐
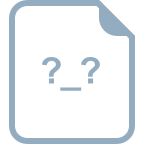
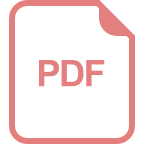





