请用go语言编写BP神经网络代码
时间: 2023-03-19 15:23:30 浏览: 194
好的,以下是一个简单的基于Go语言的BP神经网络代码示例:
```
package main
import (
"fmt"
"math"
"math/rand"
)
type Neuron struct {
weights []float64
bias float64
delta float64
output float64
}
type Layer struct {
neurons []*Neuron
}
type Network struct {
layers []*Layer
}
func sigmoid(x float64) float64 {
return 1.0 / (1.0 + math.Exp(-x))
}
func (n *Neuron) Calculate(inputs []float64) float64 {
sum := n.bias
for i, input := range inputs {
sum += input * n.weights[i]
}
n.output = sigmoid(sum)
return n.output
}
func (n *Neuron) CalculateOutputDelta(expected float64) {
error := expected - n.output
n.delta = n.output * (1.0 - n.output) * error
}
func (n *Neuron) CalculateHiddenDelta(nextLayer *Layer, index int) {
var sum float64
for _, neuron := range nextLayer.neurons {
sum += neuron.weights[index] * neuron.delta
}
n.delta = n.output * (1.0 - n.output) * sum
}
func (n *Neuron) UpdateWeights(inputs []float64, learningRate float64) {
for i, input := range inputs {
n.weights[i] += learningRate * n.delta * input
}
n.bias += learningRate * n.delta
}
func (l *Layer) Calculate(inputs []float64) []float64 {
outputs := make([]float64, len(l.neurons))
for i, neuron := range l.neurons {
outputs[i] = neuron.Calculate(inputs)
}
return outputs
}
func (l *Layer) CalculateDeltas(expected []float64, nextLayer *Layer) {
if nextLayer == nil {
for i, neuron := range l.neurons {
neuron.CalculateOutputDelta(expected[i])
}
} else {
for i, neuron := range l.neurons {
neuron.CalculateHiddenDelta(nextLayer, i)
}
}
}
func (l *Layer) UpdateWeights(inputs []float64, learningRate float64) {
for _, neuron := range l.neurons {
neuron.UpdateWeights(inputs, learningRate)
}
}
func NewNeuron(numInputs int) *Neuron {
weights := make([]float64, numInputs)
for i := range weights {
weights[i] = rand.Float64()
}
bias := rand.Float64()
return &Neuron{weights: weights, bias: bias}
}
func NewLayer(numNeurons int, numInputs int) *Layer {
neurons := make([]*Neuron, numNeurons)
for i := range neurons {
neurons[i] = NewNeuron(numInputs)
}
return &Layer{neurons: neurons}
}
func NewNetwork(numInputs, numOutputs, numHidden int) *Network {
layers := make([]*Layer, 0)
inputLayer := NewLayer(numInputs, 0)
layers = append(layers, inputLayer)
hiddenLayer := NewLayer(numHidden, numInputs)
layers = append(layers, hiddenLayer)
outputLayer := NewLayer(numOutputs, numHidden)
layers = append(layers, outputLayer)
return &Network{layers: layers}
}
func (n *Network) FeedForward(inputs []float64) []float64 {
outputs := inputs
for _,
阅读全文
相关推荐
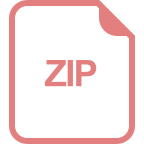
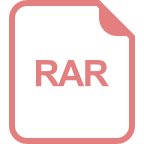
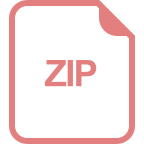















