tf.expand_dims对应torch
时间: 2023-08-31 13:08:29 浏览: 48
tf.expand_dims对应的是torch.unsqueeze函数。两个函数的作用都是在指定的维度上增加一个维度。举个例子,假设一个Tensor的shape是(3,4),那么使用tf.expand_dims或torch.unsqueeze在维度1上增加一个维度后,新的Tensor的shape都会变成(3,1,4)。具体用法如下:
TensorFlow中的tf.expand_dims用法:
```python
import tensorflow as tf
x = tf.constant([[1, 2], [3, 4]])
x = tf.expand_dims(x, 0) # 增加维度0
print(x.shape) # 输出 (1, 2, 2)
```
PyTorch中的torch.unsqueeze用法:
```python
import torch
x = torch.tensor([[1, 2], [3, 4]])
x = torch.unsqueeze(x, 0) # 增加维度0
print(x.shape) # 输出 torch.Size([1, 2, 2])
```
相关问题
讲一下tensorflow代码转为pytorch代码。def sliceup(inputs,patch_size = 3): #1->9 batch_size, h, w = inputs.shape #b,h,w,1 slice_img = inputs[:,0::3, 0::3] slice_img = tf.expand_dims(slice_img,axis=-1) for i in range(patch_size**2-1): x = (i+1) % 3 y = (i+1) // 3 temp_img = inputs[:,x::3, y::3] temp_img = tf.expand_dims(temp_img,axis=-1) slice_img = tf.concat([slice_img,temp_img],axis=-1) return slice_img #b,170,170,9
将 TensorFlow 代码转换为 PyTorch 代码需要注意以下几个方面:
1. 张量的创建方法:TensorFlow 中的张量创建方法为 tf.constant、tf.Variable 等,而 PyTorch 中的张量创建方法为 torch.tensor、torch.zeros、torch.ones 等。
2. 张量的形状:TensorFlow 中的张量形状使用 tuple 表示,例如 inputs.shape,而 PyTorch 中的张量形状使用张量的 shape 属性,例如 inputs.shape。
3. 张量的切片:TensorFlow 中的张量切片使用类似于 Python 列表切片的方式,例如 inputs[:,0::3, 0::3],而 PyTorch 中的张量切片使用类似于 NumPy 数组切片的方式,例如 inputs[:,::3, ::3]。
下面是将 TensorFlow 代码转换为 PyTorch 代码的示例:
```
import torch
def sliceup(inputs, patch_size=3):
batch_size, h, w = inputs.shape
slice_img = inputs[:, ::3, ::3].unsqueeze(-1)
for i in range(patch_size**2-1):
x = (i+1) % 3
y = (i+1) // 3
temp_img = inputs[:, x::3, y::3].unsqueeze(-1)
slice_img = torch.cat([slice_img, temp_img], axis=-1)
return slice_img
```
在 PyTorch 中,可以使用 unsqueeze 方法在指定的维度上增加一个新的维度,例如 inputs.unsqueeze(-1) 表示在最后一维上增加一个新的维度。PyTorch 中的张量切片使用类似于 NumPy 数组切片的方式,例如 inputs[:,::3, ::3] 表示按步长为 3 切片。在 PyTorch 中,可以使用 cat 方法沿着指定的维度拼接张量,例如 torch.cat([slice_img, temp_img], axis=-1) 表示在最后一维上拼接两个张量。
class CAM_Module(Module): """ Channel attention module""" def __init__(self, in_dim): super(CAM_Module, self).__init__() self.chanel_in = in_dim self.gamma = Parameter(torch.zeros(1)) self.softmax = Softmax(dim=-1) def forward(self,x): """ inputs : x : input feature maps( B X C X H X W) returns : out : attention value + input feature attention: B X C X C """ m_batchsize, C, height, width, channle = x.size() #print(x.size()) proj_query = x.view(m_batchsize, C, -1) proj_key = x.view(m_batchsize, C, -1).permute(0, 2, 1) #形状转换并交换维度 energy = torch.bmm(proj_query, proj_key) energy_new = torch.max(energy, -1, keepdim=True)[0].expand_as(energy)-energy attention = self.softmax(energy_new) proj_value = x.view(m_batchsize, C, -1) out = torch.bmm(attention, proj_value) out = out.view(m_batchsize, C, height, width, channle) # print('out', out.shape) # print('x', x.shape) out = self.gamma*out + x #C*H*W return out 将其改成keras代码
下面是将该 Channel Attention Module 的 PyTorch 代码转换为 Keras 代码的实现:
```python
from keras.layers import Layer, Softmax
import tensorflow as tf
class CAM_Module(Layer):
""" Channel attention module"""
def __init__(self, in_dim):
super(CAM_Module, self).__init__()
self.chanel_in = in_dim
self.gamma = self.add_weight(name='gamma', shape=[1], initializer='zeros', trainable=True)
self.softmax = Softmax(axis=-1)
def call(self, x):
"""
inputs :
x : input feature maps( B X H X W X C)
returns :
out : attention value + input feature
attention: B X C X C
"""
m_batchsize, height, width, C = x.shape.as_list()
# print(x.shape)
proj_query = tf.reshape(x, [-1, height*width, C])
proj_key = tf.transpose(proj_query, perm=[0, 2, 1])
energy = tf.matmul(proj_query, proj_key)
energy_new = tf.expand_dims(tf.reduce_max(energy, axis=-1), axis=-1) - energy
attention = self.softmax(energy_new)
proj_value = tf.reshape(x, [-1, height*width, C])
out = tf.matmul(attention, proj_value)
out = tf.reshape(out, [-1, height, width, C])
out = self.gamma * out + x
return out
```
注意事项:
1. Keras 中的 Layer 类相当于 PyTorch 中的 Module 类;
2. Keras 中的 add_weight 方法可以添加模型参数,相当于 PyTorch 中的 Parameter 类;
3. Keras 中的 Softmax 层在调用时需要指定 `axis` 参数,相当于 PyTorch 中的 dim 参数;
4. Keras 中的 tf.reshape 方法可以替代 PyTorch 中的 view 方法;
5. Keras 中的 tf.transpose 方法可以替代 PyTorch 中的 permute 方法;
6. Keras 中的 tf.matmul 方法可以替代 PyTorch 中的 torch.bmm 方法。
相关推荐
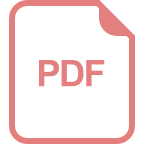
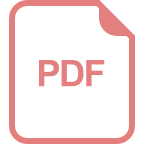
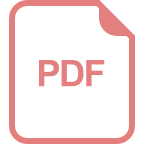











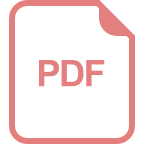